struct Win32
2
{
3
public const string TOOLBARCLASSNAME = "ToolbarWindow32";
4
5
public const int WS_CHILD = 0x40000000;
6
public const int WS_VISIBLE = 0x10000000;
7
public const int WS_CLIPCHILDREN = 0x2000000;
8
public const int WS_CLIPSIBLINGS = 0x4000000;
9
public const int WS_BORDER = 0x800000;
10
11
public const int CCS_NODIVIDER = 0x40;
12
public const int CCS_NORESIZE = 0x4;
13
public const int CCS_NOPARENTALIGN = 0x8;
14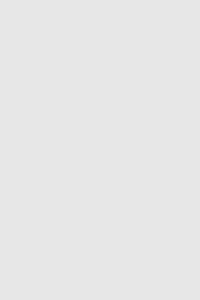
15
public const int I_IMAGECALLBACK = -1;
16
public const int I_IMAGENONE = -2;
17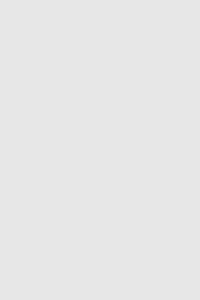
18
public const int TBSTYLE_TOOLTIPS = 0x100;
19
public const int TBSTYLE_FLAT = 0x800;
20
public const int TBSTYLE_LIST = 0x1000;
21
public const int TBSTYLE_TRANSPARENT = 0x8000;
22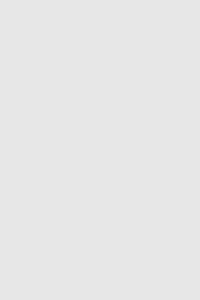
23
public const int TBSTYLE_EX_DRAWDDARROWS = 0x1;
24
public const int TBSTYLE_EX_HIDECLIPPEDBUTTONS = 0x10;
25
public const int TBSTYLE_EX_DOUBLEBUFFER = 0x80;
26
27
public const int CDRF_DODEFAULT = 0x0;
28
public const int CDRF_SKIPDEFAULT = 0x4;
29
public const int CDRF_NOTIFYITEMDRAW = 0x20;
30
public const int CDDS_PREPAINT = 0x1;
31
public const int CDDS_ITEM = 0x10000;
32
public const int CDDS_ITEMPREPAINT = CDDS_ITEM | CDDS_PREPAINT;
33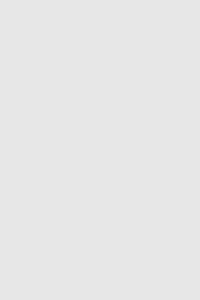
34
public const int CDIS_HOT = 0x40;
35
public const int CDIS_SELECTED = 0x1;
36
public const int CDIS_DISABLED = 0x4;
37
38
public const int WM_SETREDRAW = 0x000B;
39
public const int WM_CANCELMODE = 0x001F;
40
public const int WM_NOTIFY = 0x4e;
41
public const int WM_KEYDOWN = 0x100;
42
public const int WM_KEYUP = 0x101;
43
public const int WM_CHAR = 0x0102;
44
public const int WM_SYSKEYDOWN = 0x104;
45
public const int WM_SYSKEYUP = 0x105;
46
public const int WM_COMMAND = 0x111;
47
public const int WM_MENUCHAR = 0x120;
48
public const int WM_MOUSEMOVE = 0x200;
49
public const int WM_LBUTTONDOWN = 0x201;
50
public const int WM_MOUSELAST = 0x20a;
51
public const int WM_USER = 0x0400;
52
public const int WM_REFLECT = WM_USER + 0x1c00;
53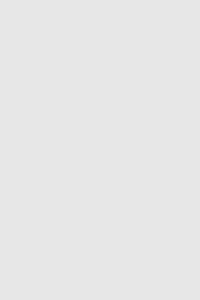
54
public const int NM_CUSTOMDRAW = -12;
55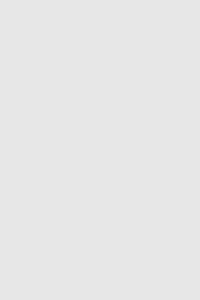
56
public const int TTN_NEEDTEXTA = ((0-520)-0);
57
public const int TTN_NEEDTEXTW = ((0-520)-10);
58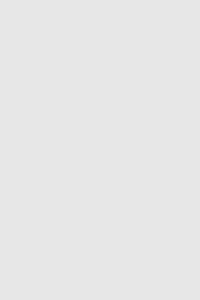
59
public const int TBN_QUERYINSERT = ((0-700)-6);
60
public const int TBN_DROPDOWN = ((0-700)-10);
61
public const int TBN_HOTITEMCHANGE = ((0 - 700) - 13);
62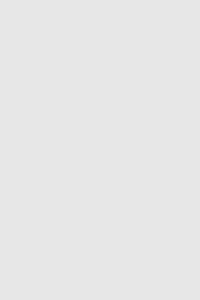
63
public const int TBIF_IMAGE = 0x1;
64
public const int TBIF_TEXT = 0x2;
65
public const int TBIF_STATE = 0x4;
66
public const int TBIF_STYLE = 0x8;
67
public const int TBIF_COMMAND = 0x20;
68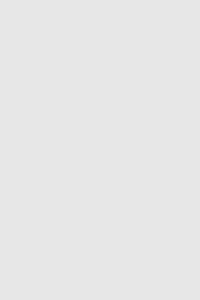
69
public const int MNC_EXECUTE = 2;
70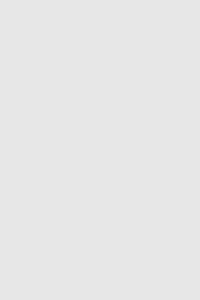
71
[StructLayout(LayoutKind.Sequential, Pack = 1)]
72
public class INITCOMMONCONTROLSEX
73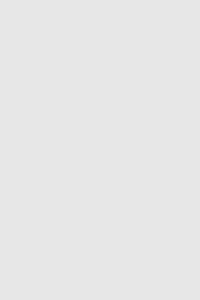
{
74
public int dwSize = 8;
75
public int dwICC;
76
}
77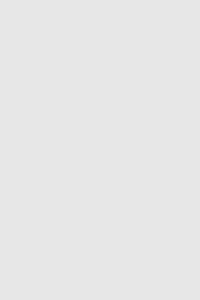
78
public const int ICC_BAR_CLASSES = 4;
79
public const int ICC_COOL_CLASSES = 0x400;
80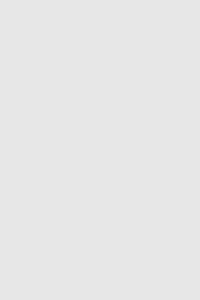
81
[DllImport("comctl32.dll")]
82
public static extern bool InitCommonControlsEx(INITCOMMONCONTROLSEX icc);
83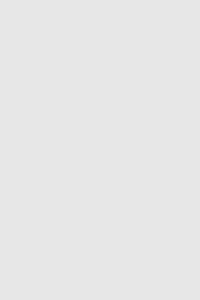
84
[StructLayout(LayoutKind.Sequential)]
85
public struct POINT
86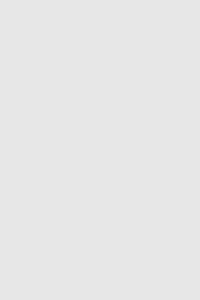
{
87
public int x;
88
public int y;
89
}
90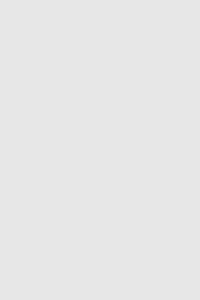
91
[StructLayout(LayoutKind.Sequential)]
92
public struct RECT
93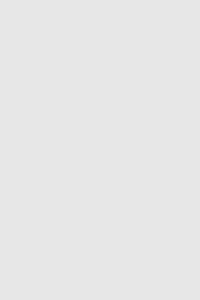
{
94
public int left;
95
public int top;
96
public int right;
97
public int bottom;
98
}
99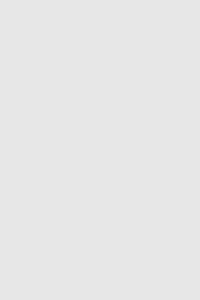
100
[StructLayout(LayoutKind.Sequential)]
101
public struct NMHDR
102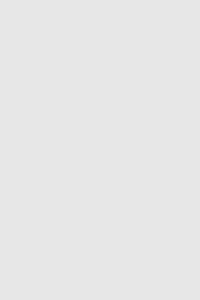
{
103
public IntPtr hwndFrom;
104
public int idFrom;
105
public int code;
106
}
107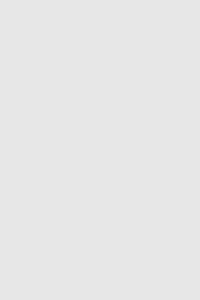
108
[StructLayout(LayoutKind.Sequential)]
109
public struct NMTOOLBAR
110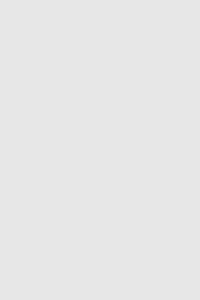
{
111
public NMHDR hdr;
112
public int iItem;
113
public TBBUTTON tbButton;
114
public int cchText;
115
public IntPtr pszText;
116
}
117
118
[StructLayout(LayoutKind.Sequential)]
119
public struct NMCUSTOMDRAW
120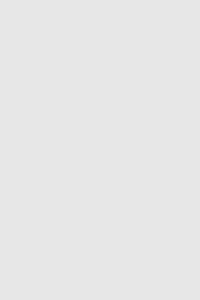
{
121
public NMHDR hdr;
122
public int dwDrawStage;
123
public IntPtr hdc;
124
public RECT rc;
125
public int dwItemSpec;
126
public int uItemState;
127
public int lItemlParam;
128
}
129
130
[StructLayout(LayoutKind.Sequential)]
131
public struct LPNMTBCUSTOMDRAW
132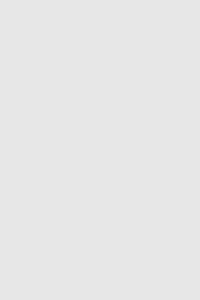
{
133
public NMCUSTOMDRAW nmcd;
134
public IntPtr hbrMonoDither;
135
public IntPtr hbrLines;
136
public IntPtr hpenLines;
137
public int clrText;
138
public int clrMark;
139
public int clrTextHighlight;
140
public int clrBtnFace;
141
public int clrBtnHighlight;
142
public int clrHighlightHotTrack;
143
public RECT rcText;
144
public int nStringBkMode;
145
public int nHLStringBkMode;
146
}
147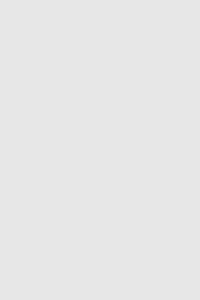
148
public const int TTF_RTLREADING = 0x0004;
149
150
[StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
151
public struct TOOLTIPTEXT
152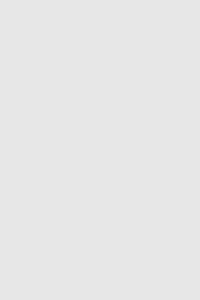
{
153
public NMHDR hdr;
154
public IntPtr lpszText;
155
[MarshalAs(UnmanagedType.ByValTStr, SizeConst=80)]
156
public string szText;
157
public IntPtr hinst;
158
public int uFlags;
159
}
160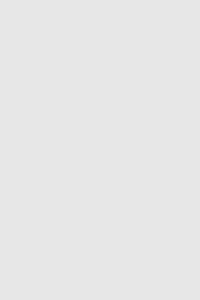
161
[StructLayout(LayoutKind.Sequential, CharSet=CharSet.Ansi)]
162
public struct TOOLTIPTEXTA
163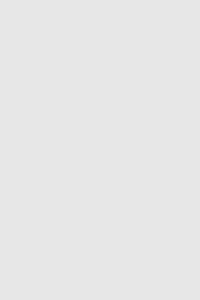
{
164
public NMHDR hdr;
165
public IntPtr lpszText;
166
[MarshalAs(UnmanagedType.ByValTStr, SizeConst=80)]
167
public string szText;
168
public IntPtr hinst;
169
public int uFlags;
170
}
171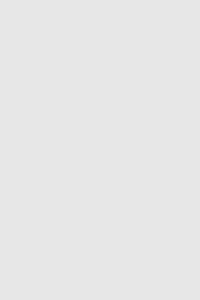
172
public const int TB_PRESSBUTTON = WM_USER + 3;
173
public const int TB_INSERTBUTTON = WM_USER + 21;
174
public const int TB_BUTTONCOUNT = WM_USER + 24;
175
public const int TB_GETITEMRECT = WM_USER + 29;
176
public const int TB_BUTTONSTRUCTSIZE = WM_USER + 30;
177
public const int TB_SETBUTTONSIZE = WM_USER + 32;
178
public const int TB_SETIMAGELIST = WM_USER + 48;
179
public const int TB_GETRECT = WM_USER + 51;
180
public const int TB_SETBUTTONINFO = WM_USER + 64;
181
public const int TB_HITTEST = WM_USER +69;
182
public const int TB_GETHOTITEM = WM_USER + 71;
183
public const int TB_SETHOTITEM = WM_USER + 72;
184
public const int TB_SETEXTENDEDSTYLE = WM_USER + 84;
185
186
public const int TBSTATE_CHECKED = 0x01;
187
public const int TBSTATE_ENABLED = 0x04;
188
public const int TBSTATE_HIDDEN = 0x08;
189
190
public const int BTNS_BUTTON = 0;
191
public const int BTNS_SEP = 0x1;
192
public const int BTNS_DROPDOWN = 0x8;
193
public const int BTNS_AUTOSIZE = 0x10;
194
public const int BTNS_WHOLEDROPDOWN = 0x80;
195
196
[StructLayout(LayoutKind.Sequential, Pack=1)]
197
public struct TBBUTTON
198
{
199
public int iBitmap;
200
public int idCommand;
201
public byte fsState;
202
public byte fsStyle;
203
public byte bReserved0;
204
public byte bReserved1;
205
public int dwData;
206
public int iString;
207
}
208
209
[StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
210
public struct TBBUTTONINFO
211
{
212
public int cbSize;
213
public int dwMask;
214
public int idCommand;
215
public int iImage;
216
public byte fsState;
217
public byte fsStyle;
218
public short cx;
219
public IntPtr lParam;
220
public IntPtr pszText;
221
public int cchText;
222
}
223
224
[DllImport("user32.dll", ExactSpelling=true, CharSet=CharSet.Auto)]
225
public static extern IntPtr GetParent(IntPtr hWnd);
226
227
[DllImport("user32.dll", CharSet=CharSet.Auto)]
228
public static extern int SendMessage(IntPtr hWnd, int msg, int wParam, int lParam);
229
230
[DllImport("user32.dll", CharSet=CharSet.Auto)]
231
public static extern IntPtr SendMessage(IntPtr hWnd, int msg, int wParam, IntPtr lParam);
232
233
[DllImport("user32.dll", CharSet=CharSet.Auto)]
234
public static extern void SendMessage(IntPtr hWnd, int msg, int wParam, ref RECT lParam);
235
236
[DllImport("user32.dll", CharSet=CharSet.Auto)]
237
public static extern int SendMessage(IntPtr hWnd, int msg, int wParam, ref POINT lParam);
238
239
[DllImport("user32.dll", CharSet=CharSet.Auto)]
240
public static extern void SendMessage(IntPtr hWnd, int msg, int wParam, ref TBBUTTON lParam);
241
242
[DllImport("user32.dll", CharSet=CharSet.Auto)]
243
public static extern void SendMessage(IntPtr hWnd, int msg, int wParam, ref TBBUTTONINFO lParam);
244
245
[DllImport("user32.dll", CharSet=CharSet.Auto)]
246
public static extern void SendMessage(IntPtr hWnd, int msg, int wParam, ref REBARBANDINFO lParam);
247
248
[DllImport("user32.dll", CharSet=CharSet.Auto)]
249
public static extern IntPtr PostMessage(IntPtr hWnd, int msg, int wParam, int lParam);
250
251
[DllImport("kernel32.dll", ExactSpelling=true, CharSet=CharSet.Auto)]
252
public static extern int GetCurrentThreadId();
253
254
public delegate IntPtr HookProc(int nCode, IntPtr wParam, IntPtr lParam);
255
256
public const int WH_MSGFILTER = -1;
257
public const int MSGF_MENU = 2;
258
259
[DllImport("user32.dll", CharSet=CharSet.Auto)]
260
public static extern IntPtr SetWindowsHookEx(int hookid, HookProc pfnhook, IntPtr hinst, int threadid);
261
262
[DllImport("user32.dll", CharSet=CharSet.Auto, ExactSpelling=true)]
263
public static extern bool UnhookWindowsHookEx(IntPtr hhook);
264
265
[DllImport("user32.dll", CharSet=CharSet.Auto, ExactSpelling=true)]
266
public static extern IntPtr CallNextHookEx(IntPtr hhook, int code, IntPtr wparam, IntPtr lparam);
267
268
[StructLayout(LayoutKind.Sequential)]
269
public struct MSG
270
{
271
public IntPtr hwnd;
272
public int message;
273
public IntPtr wParam;
274
public IntPtr lParam;
275
public int time;
276
public int pt_x;
277
public int pt_y;
278
}
279
280
public const string REBARCLASSNAME = "ReBarWindow32";
281
282
public const int RBS_VARHEIGHT = 0x200;
283
public const int RBS_BANDBORDERS = 0x400;
284
public const int RBS_AUTOSIZE = 0x2000;
285
286
public const int RBN_FIRST = -831;
287
public const int RBN_HEIGHTCHANGE = RBN_FIRST - 0;
288
public const int RBN_AUTOSIZE = RBN_FIRST - 3;
289
public const int RBN_CHEVRONPUSHED = RBN_FIRST - 10;
290
291
public const int RB_SETBANDINFO = WM_USER + 6;
292
public const int RB_GETRECT = WM_USER + 9;
293
public const int RB_INSERTBAND = WM_USER + 10;
294
public const int RB_GETBARHEIGHT = WM_USER + 27;
295
296
[StructLayout(LayoutKind.Sequential)]
297
public struct REBARBANDINFO
298
{
299
public int cbSize;
300
public int fMask;
301
public int fStyle;
302
public int clrFore;
303
public int clrBack;
304
public IntPtr lpText;
305
public int cch;
306
public int iImage;
307
public IntPtr hwndChild;
308
public int cxMinChild;
309
public int cyMinChild;
310
public int cx;
311
public IntPtr hbmBack;
312
public int wID;
313
public int cyChild;
314
public int cyMaxChild;
315
public int cyIntegral;
316
public int cxIdeal;
317
public int lParam;
318
public int cxHeader;
319
}
320
321
public const int RBBIM_CHILD = 0x10;
322
public const int RBBIM_CHILDSIZE = 0x20;
323
public const int RBBIM_STYLE = 0x1;
324
public const int RBBIM_ID = 0x100;
325
public const int RBBIM_SIZE = 0x40;
326
public const int RBBIM_IDEALSIZE = 0x200;
327
public const int RBBIM_TEXT = 0x4;
328
329
public const int RBBS_BREAK = 0x1;
330
public const int RBBS_CHILDEDGE = 0x4;
331
public const int RBBS_FIXEDBMP = 0x20;
332
public const int RBBS_GRIPPERALWAYS = 0x80;
333
public const int RBBS_USECHEVRON = 0x200;
334
335
[StructLayout(LayoutKind.Sequential)]
336
public struct NMREBARCHEVRON
337
{
338
public NMHDR hdr;
339
public int uBand;
340
public int wID;
341
public int lParam;
342
public RECT rc;
343
public int lParamNM;
344
}
345
346
[StructLayout(LayoutKind.Sequential)]
347
public struct IMAGELISTDRAWPARAMS
348
{
349
public int cbSize;
350
public IntPtr himl;
351
public int i;
352
public IntPtr hdcDst;
353
public int x;
354
public int y;
355
public int cx;
356
public int cy;
357
public int xBitmap;
358
public int yBitmap;
359
public int rgbBk;
360
public int rgbFg;
361
public int fStyle;
362
public int dwRop;
363
public int fState;
364
public int Frame;
365
public int crEffect;
366
}
367
368
public const int ILD_TRANSPARENT = 0x1;
369
public const int ILS_SATURATE = 0x4;
370
371
[DllImport("comctl32.dll", CharSet=CharSet.Auto)]
372
public static extern bool ImageList_DrawIndirect(ref IMAGELISTDRAWPARAMS pimldp);
373
374
[StructLayout(LayoutKind.Sequential)]
375
public struct DLLVERSIONINFO
376
{
377
public int cbSize;
378
public int dwMajorVersion;
379
public int dwMinorVersion;
380
public int dwBuildNumber;
381
public int dwPlatformID;
382
}
383
384
[DllImport("comctl32.dll")]
385
public extern static int DllGetVersion(ref DLLVERSIONINFO dvi);
386
387
public const int SPI_GETFLATMENU = 0x1022;
388
389
[DllImport("user32.dll", CharSet=CharSet.Auto)]
390
public extern static int SystemParametersInfo(int nAction, int nParam, ref int value, int ignore);
391
392
public const int DT_SINGLELINE = 0x20;
393
public const int DT_LEFT = 0x0;
394
public const int DT_VCENTER = 0x4;
395
public const int DT_CALCRECT = 0x400;
396
397
[DllImport("user32.dll")]
398
public extern static int DrawText(IntPtr hdc, string lpString, int nCount, ref RECT lpRect, int uFormat);
399
400
public const int TRANSPARENT = 1;
401
402
[DllImport("gdi32.dll")]
403
public extern static int SetBkMode(IntPtr hdc, int iBkMode);
404
405
[DllImport("gdi32.dll")]
406
public extern static int SetTextColor(IntPtr hdc, int crColor);
407
408
[DllImport("gdi32.dll")]
409
public extern static IntPtr SelectObject(IntPtr hdc, IntPtr hgdiobj);
410
411
[DllImport("gdi32.dll", ExactSpelling=true, CharSet=CharSet.Auto)]
412
public static extern bool DeleteObject(IntPtr hObject);
413
414
[DllImport("user32.dll", ExactSpelling=true, CharSet=CharSet.Auto)]
415
public static extern bool MessageBeep(int type);
416
417
public class TextHelper
418
{
419
public static Size GetTextSize(Graphics graphics, string text, Font font)
420
{
421
IntPtr hdc = graphics.GetHdc();
422
IntPtr fontHandle = font.ToHfont();
423
IntPtr currentFontHandle = Win32.SelectObject(hdc, fontHandle);
424
425
Win32.RECT rect = new Win32.RECT();
426
rect.left = 0;
427
rect.right = 0;
428
rect.top = 0;
429
rect.bottom = 0;
430
431
Win32.DrawText(hdc, text, text.Length, ref rect, Win32.DT_SINGLELINE | Win32.DT_LEFT | Win32.DT_CALCRECT);
432
433
Win32.SelectObject(hdc, currentFontHandle);
434
Win32.DeleteObject(fontHandle);
435
graphics.ReleaseHdc(hdc);
436
437
return new Size(rect.right - rect.left, rect.bottom - rect.top);
438
}
439
440
public static void DrawText(Graphics graphics, string text, Point point, Font font, Color color)
441
{
442
Size size = GetTextSize(graphics, text, font);
443
444
IntPtr hdc = graphics.GetHdc();
445
IntPtr fontHandle = font.ToHfont();
446
IntPtr currentFontHandle = Win32.SelectObject(hdc, fontHandle);
447
448
int currentBkMode = Win32.SetBkMode(hdc, Win32.TRANSPARENT);
449
int currentCrColor = Win32.SetTextColor(hdc, Color.FromArgb(0, color.R, color.G, color.B).ToArgb());
450
451
Win32.RECT rc = new Win32.RECT();
452
rc.left = point.X;
453
rc.top = point.Y;
454
rc.right = rc.left + size.Width;
455
rc.bottom = rc.top + size.Height;
456
457
Win32.DrawText(hdc, text, text.Length, ref rc, Win32.DT_SINGLELINE | Win32.DT_LEFT);
458
459
Win32.SetTextColor(hdc, currentCrColor);
460
Win32.SetBkMode(hdc, currentBkMode);
461
462
Win32.SelectObject(hdc, currentFontHandle);
463
Win32.DeleteObject(fontHandle);
464
graphics.ReleaseHdc(hdc);
465
}
466
}
467
468
public class ImageHelper
469
{
470
static Version version = null;
471
472
static ImageHelper()
473
{
474
Win32.DLLVERSIONINFO dvi = new Win32.DLLVERSIONINFO();
475
dvi.cbSize = Marshal.SizeOf(typeof(Win32.DLLVERSIONINFO));
476
Win32.DllGetVersion(ref dvi);
477
version = new Version(dvi.dwMajorVersion, dvi.dwMinorVersion, dvi.dwBuildNumber, 0);
478
}
479
480
public static Version Version
481
{
482
get
{ return version; }
483
}
484
485
public static void DrawImage(Graphics graphics, Image image, Point point, bool disabled)
486
{
487
if (!disabled)
488
{
489
graphics.DrawImage(image, point);
490
return;
491
}
492
493
// Painting a disabled gray scale image is done using ILS_SATURATE on WinXP.
494
// This code emulates that behaviour if comctl32 version 6 is not availble.
495
if (version.Major < 6)
496
{
497
ImageAttributes attributes = new ImageAttributes();
498
Rectangle destination = new Rectangle(point, image.Size);
499
float[][] matrix = new float[5][];
500
matrix[0] = new float[]
{ 0.2222f, 0.2222f, 0.2222f, 0.0000f, 0.0000f };
501
matrix[1] = new float[]
{ 0.2222f, 0.2222f, 0.2222f, 0.0000f, 0.0000f };
502
matrix[2] = new float[]
{ 0.2222f, 0.2222f, 0.2222f, 0.0000f, 0.0000f };
503
matrix[3] = new float[]
{ 0.3333f, 0.3333f, 0.3333f, 0.7500f, 0.0000f };
504
matrix[4] = new float[]
{ 0.0000f, 0.0000f, 0.0000f, 0.0000f, 1.0000f };
505
attributes.SetColorMatrix(new ColorMatrix(matrix));
506
graphics.DrawImage(image, destination, 0, 0, image.Width, image.Height, GraphicsUnit.Pixel, attributes);
507
}
508
else
509
{
510
ImageList imageList = new ImageList();
511
imageList.ImageSize = image.Size;
512
imageList.ColorDepth = ColorDepth.Depth32Bit;
513
imageList.Images.Add(image);
514
515
IntPtr hdc = graphics.GetHdc();
516
Win32.IMAGELISTDRAWPARAMS ildp = new Win32.IMAGELISTDRAWPARAMS();
517
ildp.cbSize = Marshal.SizeOf(typeof(Win32.IMAGELISTDRAWPARAMS));
518
ildp.himl = imageList.Handle;
519
ildp.i = 0; // image index
520
ildp.hdcDst = hdc;
521
ildp.x = point.X;
522
ildp.y = point.Y;
523
ildp.cx = 0;
524
ildp.cy = 0;
525
ildp.xBitmap = 0;
526
ildp.yBitmap = 0;
527
ildp.fStyle = Win32.ILD_TRANSPARENT;
528
ildp.fState = Win32.ILS_SATURATE;
529
ildp.Frame = -100;
530
Win32.ImageList_DrawIndirect(ref ildp);
531
graphics.ReleaseHdc(hdc);
532
return;
533
}
534
}
535
}
536
}