一.上传图片到数据库
1 /**//// <summary> 2 /// 上传图片 3 /// </summary> 4 private void UploadFile() 5  { 6 /**////得到用户要上传的文件名 7 string strFilePathName = loFile.PostedFile.FileName; 8 string strFileName = Path.GetFileName(strFilePathName); 9 int FileLength = loFile.PostedFile.ContentLength; 10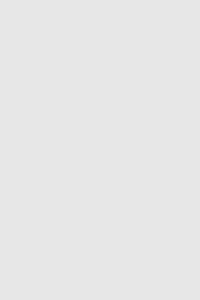 11 if(FileLength<=0) 12 return; 13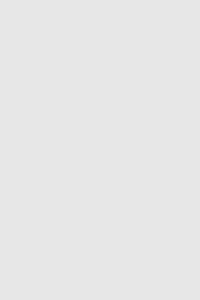 14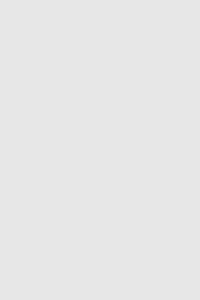 /**////上传文件 15 try 16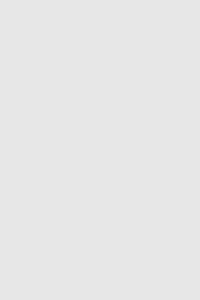 { 17 18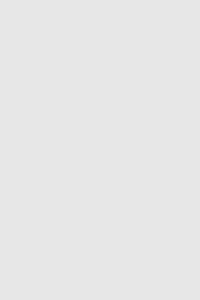 /**////图象文件临时储存Byte数组 19 Byte[] FileByteArray = new Byte[FileLength]; 20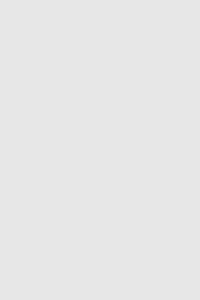 21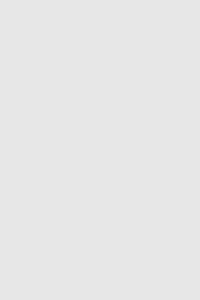 /**////建立数据流对像 22 Stream StreamObject = loFile.PostedFile.InputStream; 23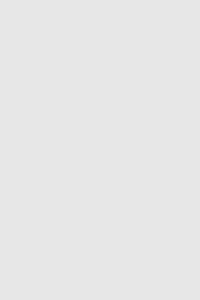 24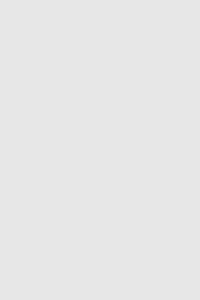 /**////读取图象文件数据,FileByteArray为数据储存体,0为数据指针位置、FileLnegth为数据长度 25 StreamObject.Read(FileByteArray,0,FileLength); 26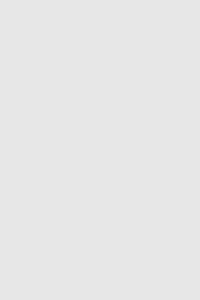 27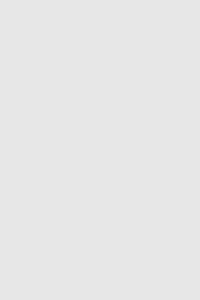 /**////建立SQL Server链接 28 string strCon = System.Configuration.ConfigurationSettings.AppSettings["DSN"]; 29 SqlConnection Con = new SqlConnection(strCon); 30 String SqlCmd = "INSERT INTO ImageStore (ImageData, ImageContentType, ImageDescription, ImageSize) VALUES (@Image, @ContentType, @ImageDescription, @ImageSize)"; 31 SqlCommand CmdObj = new SqlCommand(SqlCmd, Con); 32 CmdObj.Parameters.Add("@Image",SqlDbType.Binary, FileLength).Value = FileByteArray; 33 CmdObj.Parameters.Add("@ContentType", SqlDbType.VarChar,50).Value = loFile.PostedFile.ContentType; //记录文件类型 34 35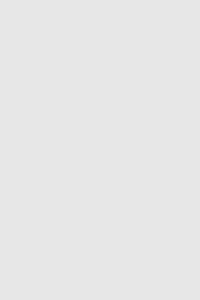 /**////把其它单表数据记录上传 36 CmdObj.Parameters.Add("@ImageDescription", SqlDbType.VarChar,200).Value = tbDescription.Text; 37 38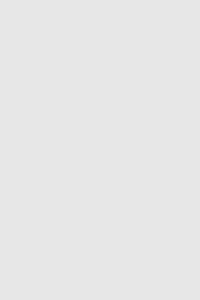 /**////记录文件长度,读取时使用 39 CmdObj.Parameters.Add("@ImageSize", SqlDbType.BigInt,8).Value = FileLength; 40 Con.Open(); 41 CmdObj.ExecuteNonQuery(); 42 Con.Close(); 43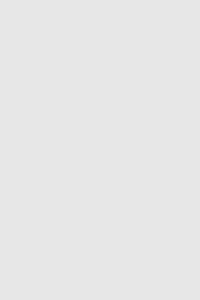 44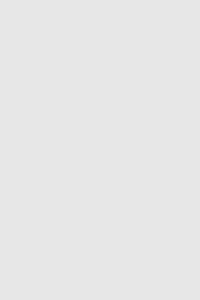 /**////跳转页面 45 Response.Redirect("ShowAll.aspx"); 46 } 47 catch(Exception ex) 48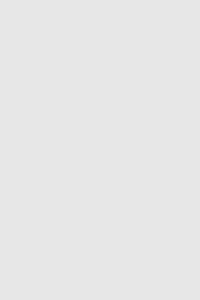 { 49 throw ex; 50 } 51 }
二.从数据库中读取图片
1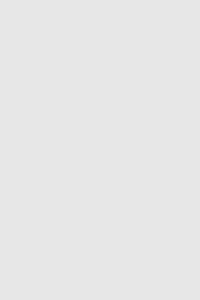 /**//// <summary> 2 /// 显示图片 3 /// </summary> 4 private void ShowImages() 5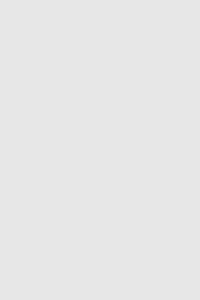 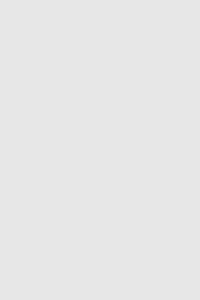 { 6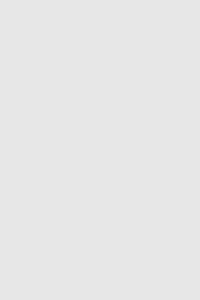 /**////ID为图片ID 7 int ImgID = Convert.ToInt32(Request.QueryString["ID"]); 8 9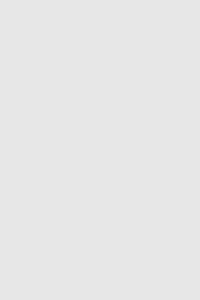 /**////建立数据库连接 10 string strCon = System.Configuration.ConfigurationSettings.AppSettings["DSN"]; 11 SqlConnection Con = new SqlConnection(strCon); 12 String SqlCmd = "SELECT * FROM ImageStore WHERE ImageID = @ImageID"; 13 SqlCommand CmdObj = new SqlCommand(SqlCmd, Con); 14 CmdObj.Parameters.Add("@ImageID", SqlDbType.Int).Value = ImgID; 15 16 Con.Open(); 17 SqlDataReader SqlReader = CmdObj.ExecuteReader(); 18 SqlReader.Read(); 19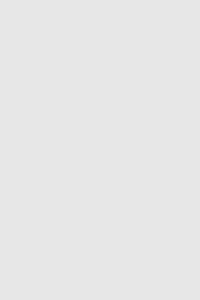 20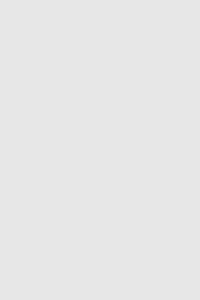 /**////设定输出文件类型 21 Response.ContentType = (string)SqlReader["ImageContentType"]; 22 23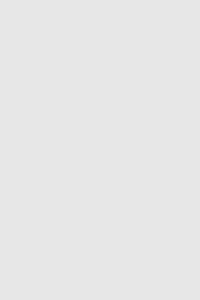 /**////输出图象文件二进制数制 24 Response.OutputStream.Write((byte[])SqlReader["ImageData"], 0, (int)SqlReader["ImageSize"]); 25 Response.End(); 26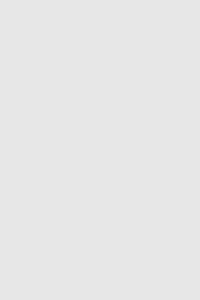 27 Con.Close(); 28 }
|