using System;

using System.Configuration;

using System.IO;

using System.Web;


namespace SingingEels
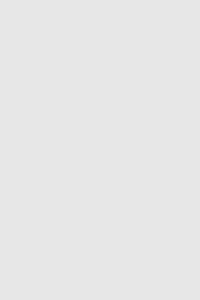
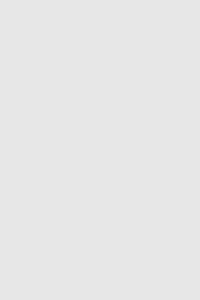 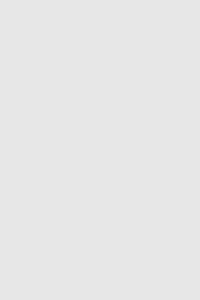 {
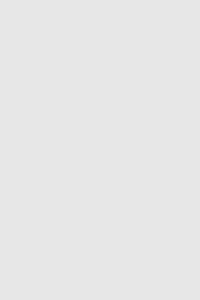
public class ErrorLogger
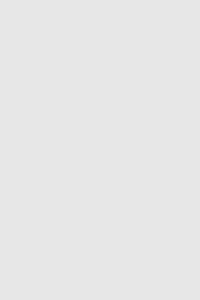
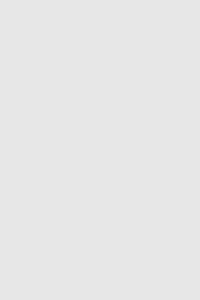 {
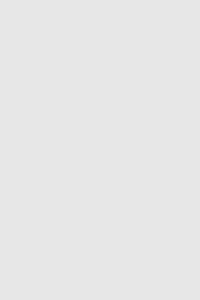
public static void LogException(Exception ex)
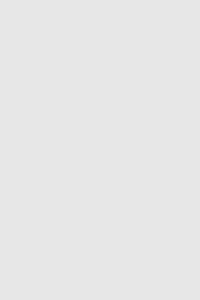
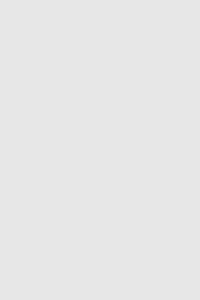 {
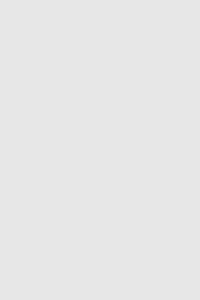
ErrorLoggerSection configInstance = ConfigurationManager.GetSection("WebSiteErrorLogger") as ErrorLoggerSection;
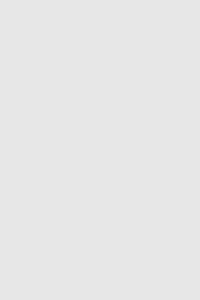
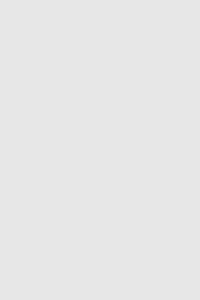
if (configInstance != null)
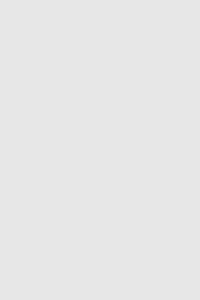
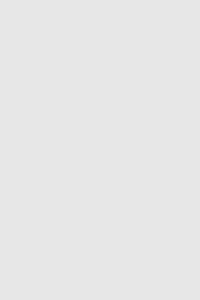 {
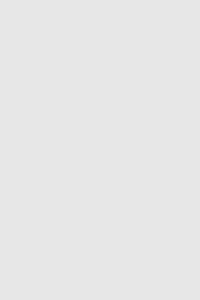
string myDirectory=HttpContext.Current.Server.MapPath(configInstance.LogFilePath + DateTime.Now.Year.ToString()+"/"+ DateTime.Now.Month.ToString()+"/");
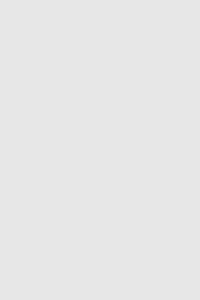
string mypath=myDirectory+DateTime.Now.ToShortDateString()+".txt";
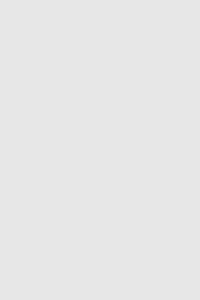
if (!Directory.Exists(myDirectory))
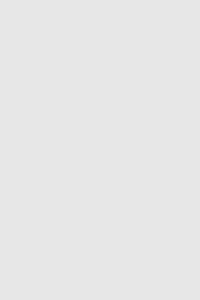
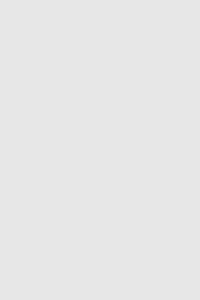 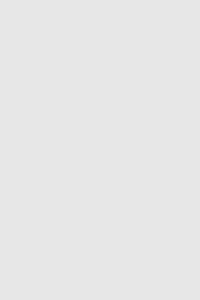 {
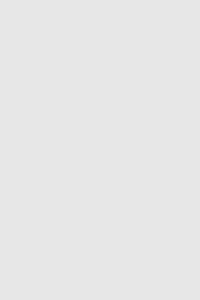
Directory.CreateDirectory(myDirectory);
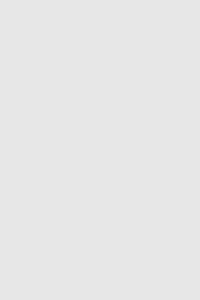
}
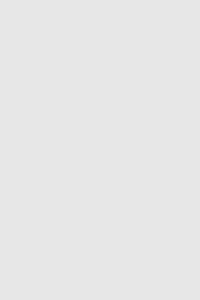
File.AppendAllText(mypath, string.Format("{0:yyyyMMdd_HHmmss} :: {1}{2}{2}", DateTime.Now, ex, Environment.NewLine));
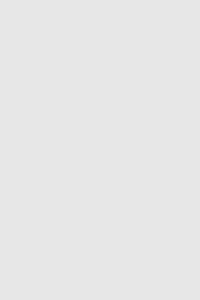
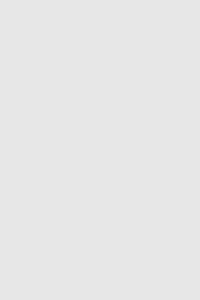
}
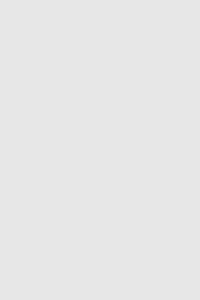
else
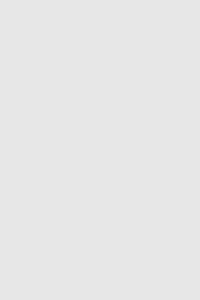
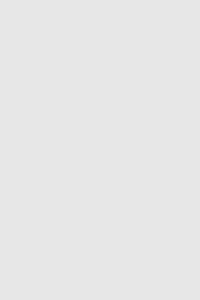 {
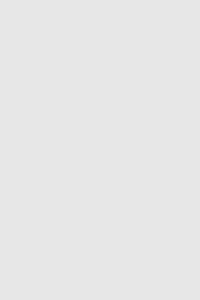
throw new Exception("Could not find section ‘ErrorLoggerSection‘ in the application configuration file.");
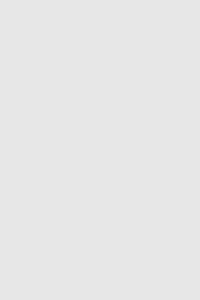
}
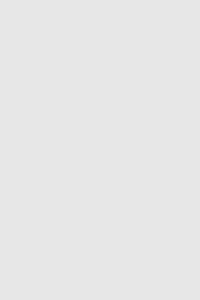
}
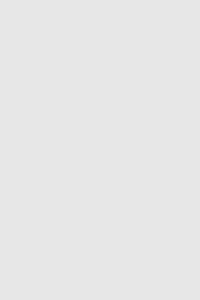
}
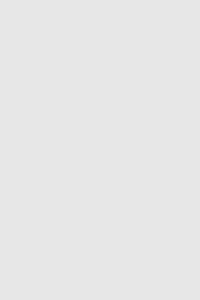
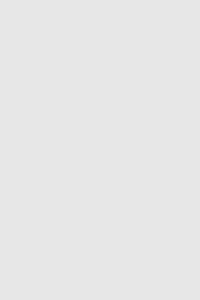
// Inherit from "ConfigurationSection" simple enough!
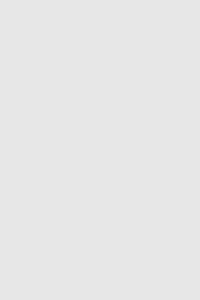
public class ErrorLoggerSection : ConfigurationSection
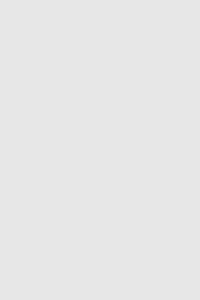
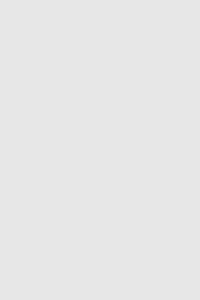 {
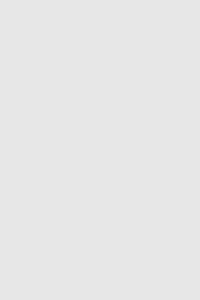
// I only want one property LogFilePath, and I‘m
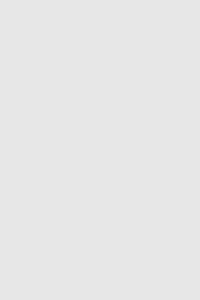
// here telling .NET to look for an attribute in the
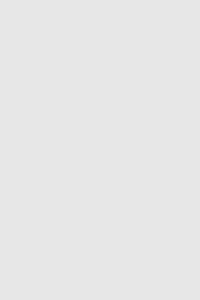
// config file called ‘logFilePath‘ to get the value from.
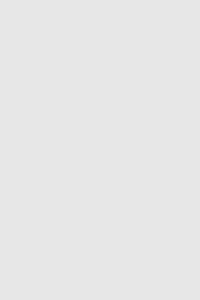
[ConfigurationProperty("logFilePath", IsRequired = true)]
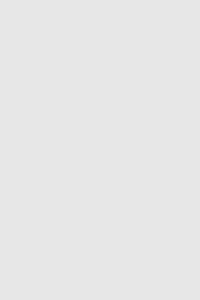
public string LogFilePath
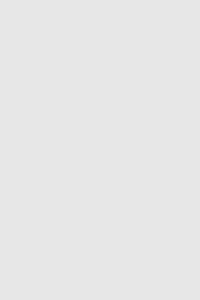
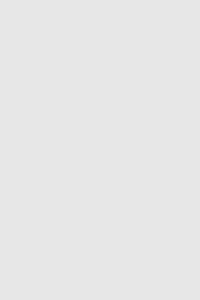 {
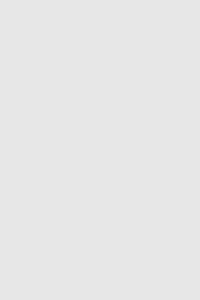
get
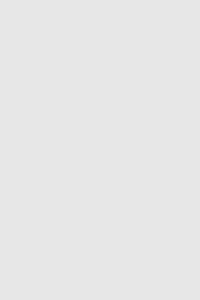
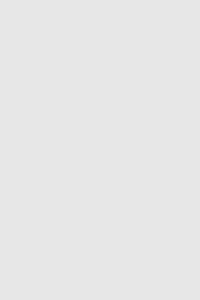 {
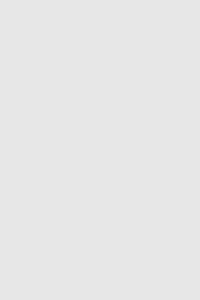
return (string)base["logFilePath"];
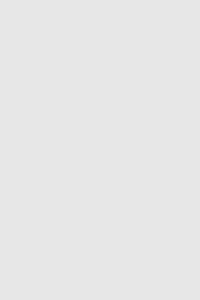
}
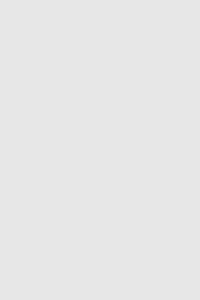
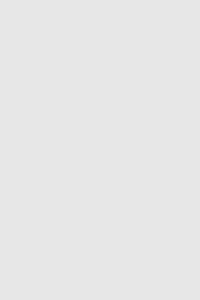
set
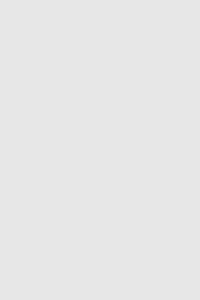
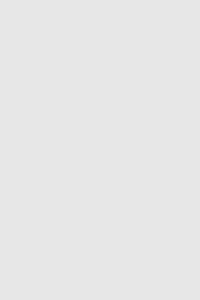 {
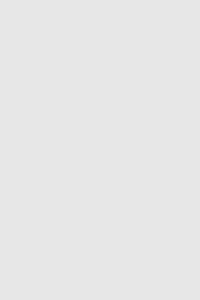
base["logFilePath"] = value;
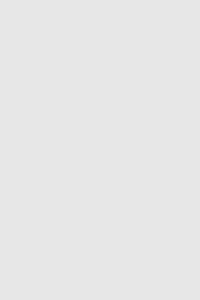
}
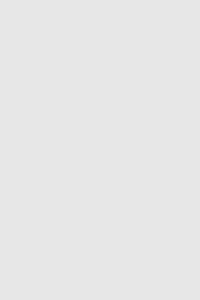
}
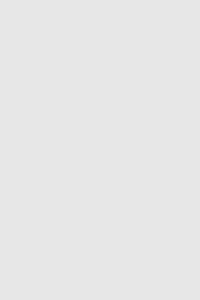
}
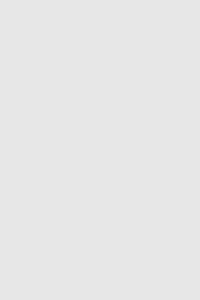
}
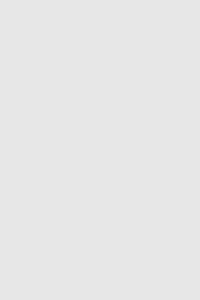
Web.config
<?xml version="1.0"?>
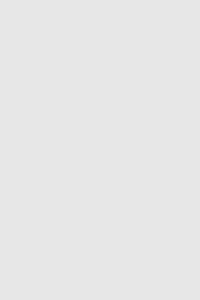
<configuration>
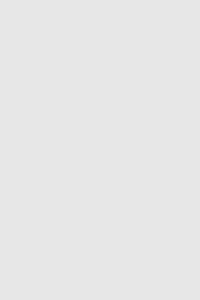
<configSections>
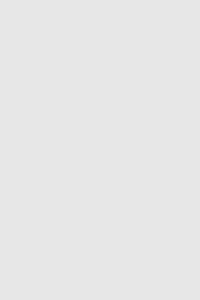
<section name="WebSiteErrorLogger" type="SingingEels.ErrorLoggerSection"/>
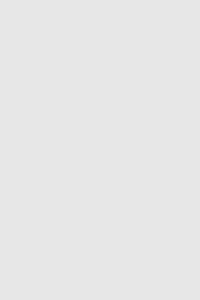
</configSections>
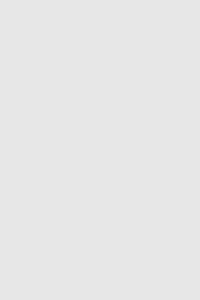
<WebSiteErrorLogger logFilePath="~/Log/"/>
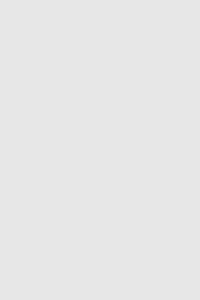
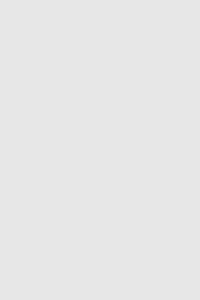
<system.web>
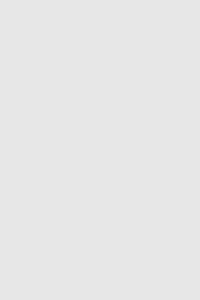
<compilation debug="true"/>
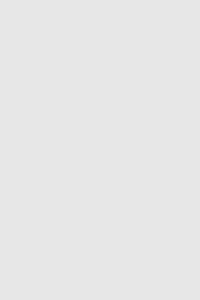
</system.web>
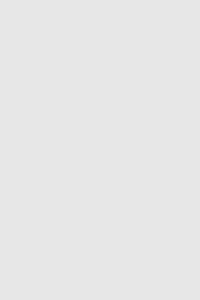
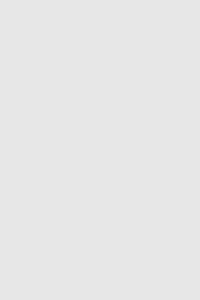
<system.web>
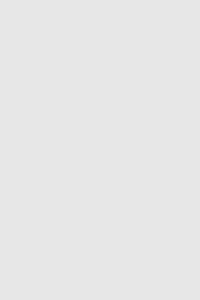
<customErrors mode="RemoteOnly" defaultRedirect="~/Error.htm"></customErrors>
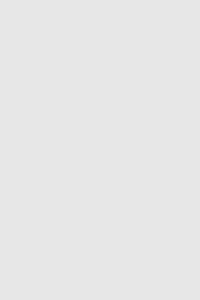
</system.web>
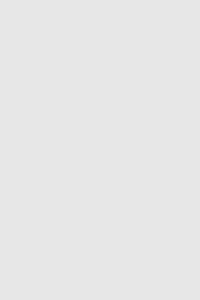
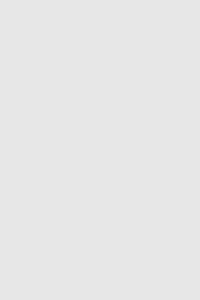
</configuration>
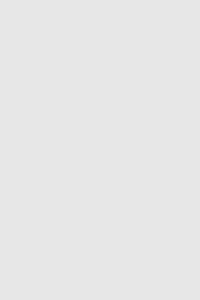
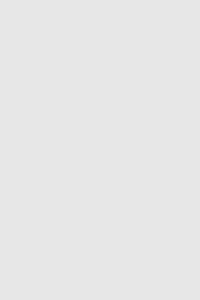
Global.asax
<%@ Application Language="C#" %>
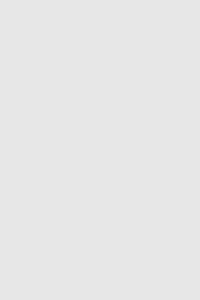
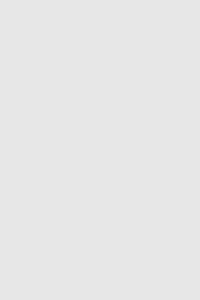
<script runat="server">
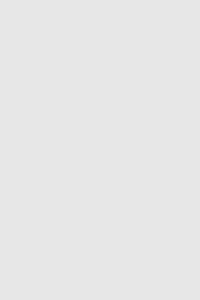
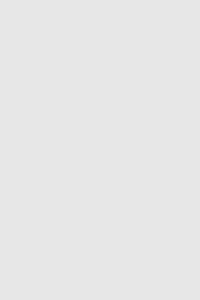
void Application_Start(object sender, EventArgs e)
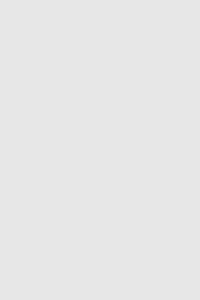
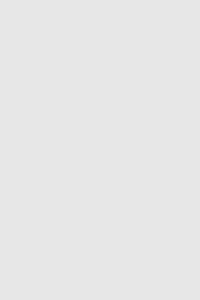 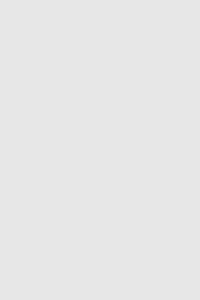 {
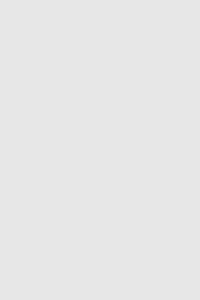
// Code that runs on application startup
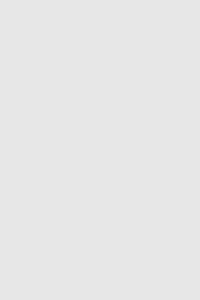
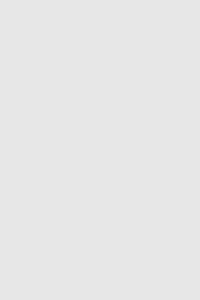
}
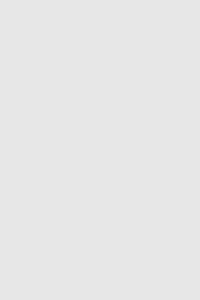
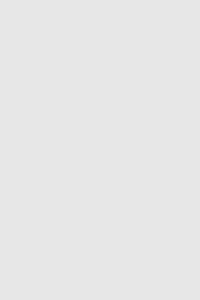
void Application_End(object sender, EventArgs e)
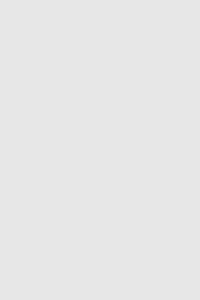
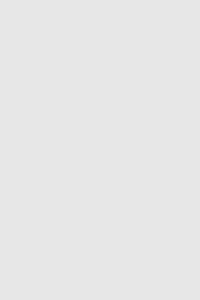 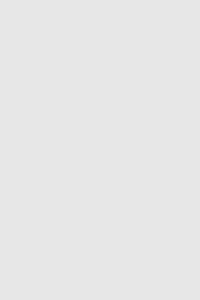 {
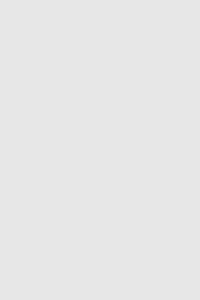
// Code that runs on application shutdown
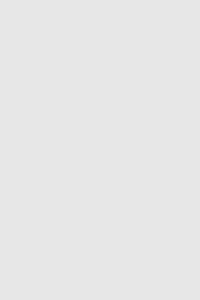
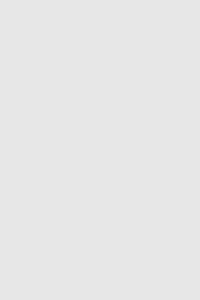
}
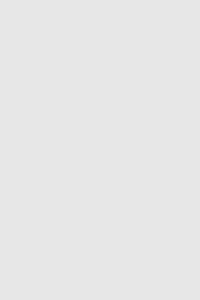
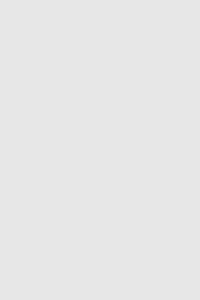
void Application_Error(object sender, EventArgs e)
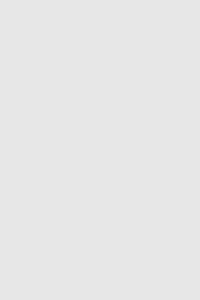
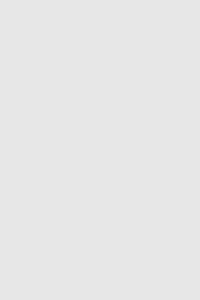 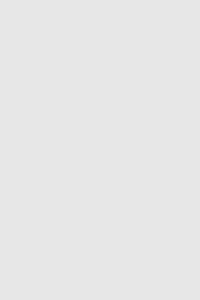 {

// Code that runs when an unhandled error occurs


Exception err = Server.GetLastError().GetBaseException();

SingingEels.ErrorLogger.LogException(err);




}


void Session_Start(object sender, EventArgs e)

  {

// Code that runs when a new session is started


}


void Session_End(object sender, EventArgs e)

  {

// Code that runs when a session ends.

// Note: The Session_End event is raised only when the sessionstate mode

// is set to InProc in the Web.config file. If session mode is set to StateServer

// or SQLServer, the event is not raised.


}


</script>
|