DataAdapter 的 Update 方法:将 DataSet 中的更改解析回数据源。DataSet保存的数据是位于服务器内存里面的原数据库的“副本”。所以用DataSet更新数据的过程就是先对“副本”进行更新,然后在将“原本”更新。
Update 方法会将更改解析回数据源,但是自上次填充 DataSet 以来,其他客户端可能已修改了数据源中的数据。若要使用当前数据刷新 DataSet,请再次使用 DataAdapter 填充 (Fill) DataSet。
EntityAA.cs
1 using System; 2 using System.Data; 3 using System.Data.SqlClient; 4 5 namespace DataSetAdapter 6  { 7 /**//// <summary> 8 /// Summary description for EntityAA. 9 /// </summary> 10 public class EntityAA 11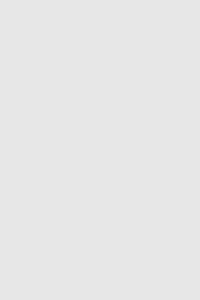 { 12 private string connstr = System.Configuration.ConfigurationSettings.AppSettings["connString"]; 13 private SqlConnection conn; 14 15 private string sql; 16 17 private SqlDataAdapter adp; 18 private SqlCommandBuilder cb; 19 20 private DataSet ds; 21 private DataTable dt; 22 23 public EntityAA() 24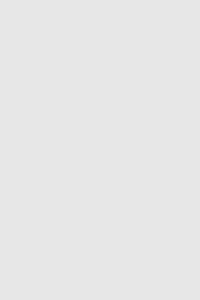 { 25 conn = new SqlConnection(connstr); 26 sql = "select * from aa"; 27 28 adp = new SqlDataAdapter(sql,conn); 29 cb = new SqlCommandBuilder(adp); 30 31 ds = new DataSet(); 32 33 FillDataSet(); 34 35 dt = ds.Tables["table_aa"]; 36 37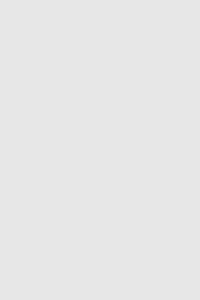 dt.PrimaryKey = new DataColumn[] {dt.Columns["a"]}; 38 } 39 40 private void FillDataSet() 41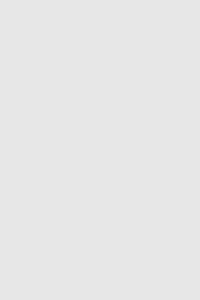 { 42 conn.Open(); 43 adp.Fill(ds,"table_aa"); 44 conn.Close(); 45 } 46 47 public DataSet List 48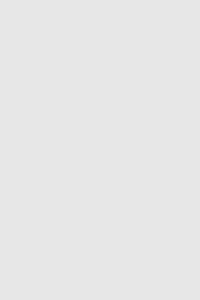 { 49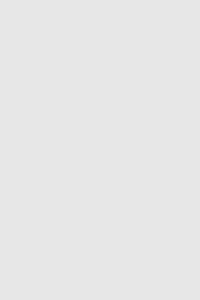 get {return ds;} 50 } 51 52 public void insert(string c) 53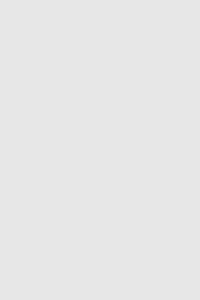 { 54 dt.Columns["a"].AutoIncrement = true; 55 56 DataRow dr = dt.NewRow(); 57 dr["c"] = c; 58 dt.Rows.Add(dr); //添加新行 59 60 adp.Update(ds,"table_aa"); 61 62 } 63 64 public void up_date(int ids,string name) 65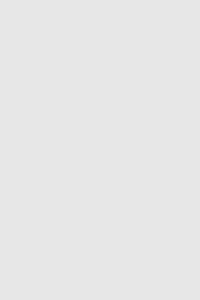 { 66 DataRow dr = dt.Rows.Find(ids); //获取由主键值指定的行 67 dr["c"] = name; //更新 68 69 adp.Update(ds,"table_aa"); 70 } 71 72 public void del(int ids) 73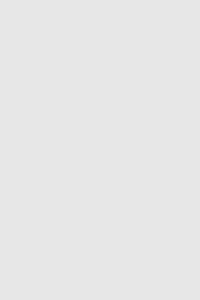 { 74 DataRow dr = dt.Rows.Find(ids); //获取由主键值指定的行 75 dr.Delete(); 76 77 adp.Update(ds,"table_aa"); 78 79 } 80 81 82 } 83 } 84
WebForm1.aspx.cs
using System;
using System.Collections;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Web;
using System.Web.SessionState;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
namespace DataSetAdapter
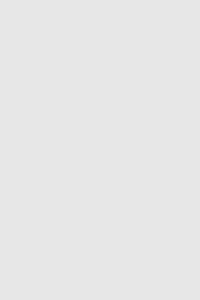 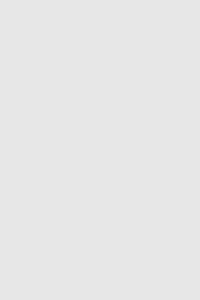 {
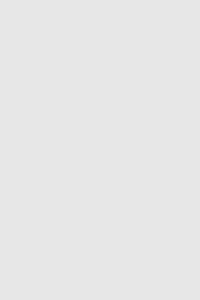 /**//// <summary>
/// Summary description for WebForm1.
/// </summary>
public class WebForm1 : System.Web.UI.Page
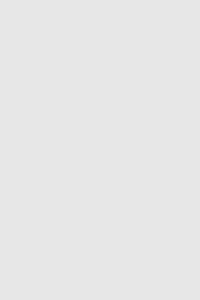 {
protected System.Web.UI.WebControls.Label Label1;
protected System.Web.UI.WebControls.Label Label2;
protected System.Web.UI.WebControls.TextBox txt_a;
protected System.Web.UI.WebControls.TextBox txt_c;
protected System.Web.UI.WebControls.Button delete;
protected System.Web.UI.WebControls.Button Button2;
protected System.Web.UI.WebControls.DataGrid DataGrid1;
protected System.Web.UI.WebControls.Button Button1;
private void Page_Load(object sender, System.EventArgs e)
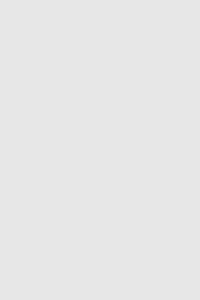 {
if(!this.Page.IsPostBack)
BindGrid();
}
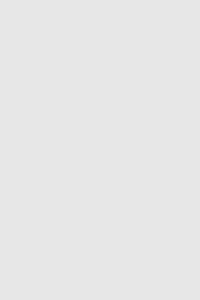 Web Form Designer generated code#region Web Form Designer generated code
override protected void OnInit(EventArgs e)
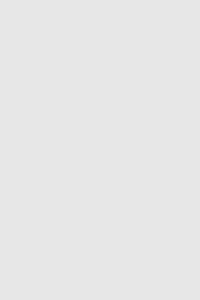 {
//
// CODEGEN: This call is required by the ASP.NET Web Form Designer.
//
InitializeComponent();
base.OnInit(e);
}
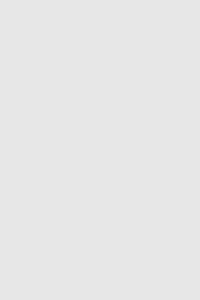 /**//// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
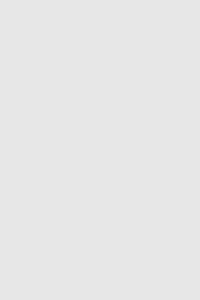 {
this.Button1.Click += new System.EventHandler(this.Button1_Click);
this.delete.Click += new System.EventHandler(this.delete_Click);
this.Button2.Click += new System.EventHandler(this.Button2_Click);
this.Load += new System.EventHandler(this.Page_Load);
}
#endregion
private void BindGrid()
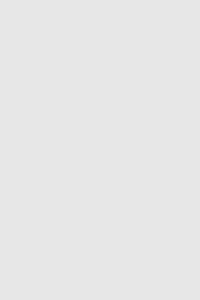 {
EntityAA entityaa = new EntityAA();
DataSet ds = entityaa.List;
this.DataGrid1.DataSource = ds;
this.DataGrid1.DataBind();
}
private void Button1_Click(object sender, System.EventArgs e)
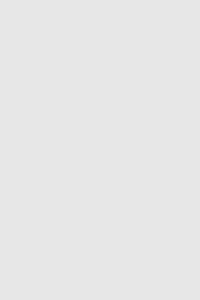 {
int ids = Int32.Parse(this.txt_a.Text);
string name = this.txt_c.Text;
EntityAA entityaa = new EntityAA();
entityaa.up_date(ids,name);
BindGrid();
}
private void delete_Click(object sender, System.EventArgs e)
 {
int ids = Int32.Parse(this.txt_a.Text);
EntityAA entityaa = new EntityAA();
entityaa.del(ids);
BindGrid();
}
private void Button2_Click(object sender, System.EventArgs e)
 {
string c = this.txt_c.Text;
EntityAA entityaa = new EntityAA();
entityaa.insert(c);
BindGrid();
}
}
}
|