设备驱动程序模型建立在几个基本数据结构上,这些结构描述了总线、设备、设备驱动、属性以及他们之间的关系。我们首先认识一下这些数据结构。 一、数据结构
设备表述符
- struct device {
- struct device *parent;
-
-
- struct device_private *p;
-
- struct kobject kobj;
- const char *init_name;
- struct device_type *type;
-
- struct semaphore sem;
-
-
-
- struct bus_type *bus;
- struct device_driver *driver;
-
- void *platform_data;
-
- struct dev_pm_info power;
-
- #ifdef CONFIG_NUMA
- int numa_node;
- #endif
- u64 *dma_mask;
- u64 coherent_dma_mask;
-
-
-
-
-
- struct device_dma_parameters *dma_parms;
-
- struct list_head dma_pools;
-
- struct dma_coherent_mem *dma_mem;
-
-
- struct dev_archdata archdata;
-
- dev_t devt;
-
- spinlock_t devres_lock;
- struct list_head devres_head;
-
- struct klist_node knode_class;
- struct class *class;
- const struct attribute_group **groups;
-
- void (*release)(struct device *dev);
- };
Bus描述符
- struct bus_type {
- const char *name;
-
- struct bus_attribute *bus_attrs;
-
- struct device_attribute *dev_attrs;
-
-
- struct driver_attribute *drv_attrs;
-
- int (*match)(struct device *dev, struct device_driver *drv);
-
- int (*uevent)(struct device *dev, struct kobj_uevent_env *env);
- int (*probe)(struct device *dev);
- int (*remove)(struct device *dev);
- void (*shutdown)(struct device *dev);
-
- int (*suspend)(struct device *dev, pm_message_t state);
- int (*resume)(struct device *dev);
-
- const struct dev_pm_ops *pm;
-
- struct bus_type_private *p;
- };
设备驱动
-
- struct device_driver {
- const char *name;
- struct bus_type *bus;
-
- struct module *owner;
- const char *mod_name;
-
- bool suppress_bind_attrs;
-
- int (*probe) (struct device *dev);
- int (*remove) (struct device *dev);
- void (*shutdown) (struct device *dev);
- int (*suspend) (struct device *dev, pm_message_t state);
- int (*resume) (struct device *dev);
- const struct attribute_group **groups;
-
- const struct dev_pm_ops *pm;
-
- struct driver_private *p;
- };
类描述符
-
-
-
- struct class {
- const char *name;
- struct module *owner;
-
- struct class_attribute *class_attrs;
- struct device_attribute *dev_attrs;
- struct kobject *dev_kobj;
-
- int (*dev_uevent)(struct device *dev, struct kobj_uevent_env *env);
- char *(*devnode)(struct device *dev, mode_t *mode);
-
- void (*class_release)(struct class *class);
- void (*dev_release)(struct device *dev);
-
- int (*suspend)(struct device *dev, pm_message_t state);
- int (*resume)(struct device *dev);
-
- const struct dev_pm_ops *pm;
-
- struct class_private *p;
- };
下面数据结构用以描述结构间关系的
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- struct device_private {
- struct klist klist_children;
- struct klist_node knode_parent;
- struct klist_node knode_driver;
- struct klist_node knode_bus;
- void *driver_data;
- struct device *device;
- };
- struct class_private {
- struct kset class_subsys;
- struct klist class_devices;
- struct list_head class_interfaces;
- struct kset class_dirs;
- struct mutex class_mutex;
- struct class *class;
- };
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- struct bus_type_private {
- struct kset subsys;
- struct kset *drivers_kset;
- struct kset *devices_kset;
- struct klist klist_devices;
- struct klist klist_drivers;
- struct blocking_notifier_head bus_notifier;
- unsigned int drivers_autoprobe:1;
- struct bus_type *bus;
- };
-
- struct driver_private {
- struct kobject kobj;
- struct klist klist_devices;
- struct klist_node knode_bus;
- struct module_kobject *mkobj;
- struct device_driver *driver;
- };
描述属性文件的结构
-
-
-
-
-
- struct attribute {
- const char *name;
- struct module *owner;
- mode_t mode;
- };
后面有时间我会补上上面数据结构之间的关系图,便于理解。
二、原理与源码解析
解决问题我还是习惯追根溯源,我们从内核启动对设备驱动模型初始化开始看起,设备驱动中sys/文件系统的初始化工作为start_kernel()->rest_init()->
- static noinline void __init_refok rest_init(void)
- __releases(kernel_lock)
- {
- ……
-
-
- kernel_thread(kernel_init, NULL, CLONE_FS | CLONE_SIGHAND);
- ……
- }
内核线程kernel_init()用于驱动的初始化
kernel_init()->do_basic_setup()->driver_init()
-
-
-
-
-
-
-
- void __init driver_init(void)
- {
-
-
-
-
-
- devtmpfs_init();
-
- devices_init();
-
- buses_init();
-
- classes_init();
-
- firmware_init();
-
-
-
-
-
-
-
- hypervisor_init();
-
-
-
-
- platform_bus_init();
-
- system_bus_init();
-
- cpu_dev_init();
-
-
-
- memory_dev_init();
- }
-
- int __init devices_init(void)
- {
-
- devices_kset = kset_create_and_add("devices", &device_uevent_ops, NULL);
- if (!devices_kset)
- return -ENOMEM;
-
- dev_kobj = kobject_create_and_add("dev", NULL);
- if (!dev_kobj)
- goto dev_kobj_err;
-
- sysfs_dev_block_kobj = kobject_create_and_add("block", dev_kobj);
- if (!sysfs_dev_block_kobj)
- goto block_kobj_err;
-
- sysfs_dev_char_kobj = kobject_create_and_add("char", dev_kobj);
- if (!sysfs_dev_char_kobj)
- goto char_kobj_err;
-
- return 0;
-
- char_kobj_err:
- kobject_put(sysfs_dev_block_kobj);
- block_kobj_err:
- kobject_put(dev_kobj);
- dev_kobj_err:
- kset_unregister(devices_kset);
- return -ENOMEM;
- }
上面代码执行后生成的目录如下图
/sys/下的dev/和devices/目录

/sys/dev/下的block/和char/目录

总线初始化
- int __init buses_init(void)
- {
-
- bus_kset = kset_create_and_add("bus", &bus_uevent_ops, NULL);
- if (!bus_kset)
- return -ENOMEM;
- return 0;
- }
运行结果图,在/sys/目录下生成/bus/目录

Class初始化
- int __init classes_init(void)
- {
-
- class_kset = kset_create_and_add("class", NULL, NULL);
- if (!class_kset)
- return -ENOMEM;
- return 0;
- }
运行结果,在/sys/目录下生成/class/目录

Firmware初始化
- int __init firmware_init(void)
- {
-
- firmware_kobj = kobject_create_and_add("firmware", NULL);
- if (!firmware_kobj)
- return -ENOMEM;
- return 0;
- }
运行结果,在/sys/目录下生成/firmware/目录

Hypervisor初始化,该功能需要内核编译选项支持,该函数需要定义CONFIG_SYS_HYPERVISOR宏,在我机器上没有编译此功能。
- int __init hypervisor_init(void)
- {
-
- hypervisor_kobj = kobject_create_and_add("hypervisor", NULL);
- if (!hypervisor_kobj)
- return -ENOMEM;
- return 0;
- }
Platform_bus初始化,下面会详细讲解
- int __init platform_bus_init(void)
- {
- int error;
-
- early_platform_cleanup();
-
- error = device_register(&platform_bus);
- if (error)
- return error;
-
- error = bus_register(&platform_bus_type);
- if (error)
- device_unregister(&platform_bus);
- return error;
- }
- struct device platform_bus = {
-
- .init_name = "platform",
- };
注册platform_bus设备
platform_bus_init()->device_register()
- int device_register(struct device *dev)
- {
- device_initialize(dev);
- return device_add(dev);
- }
设备初始化
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- void device_initialize(struct device *dev)
- {
-
- dev->kobj.kset = devices_kset;
- kobject_init(&dev->kobj, &device_ktype);
- INIT_LIST_HEAD(&dev->dma_pools);
- init_MUTEX(&dev->sem);
- spin_lock_init(&dev->devres_lock);
- INIT_LIST_HEAD(&dev->devres_head);
- device_init_wakeup(dev, 0);
- device_pm_init(dev);
- set_dev_node(dev, -1);
- }
添加设备到设备树中,我们结合实际的实现结果分析
int device_add(struct device *dev)
{
struct device *parent = NULL;
struct class_interface *class_intf;/*class接口*/
int error = -EINVAL;
dev = get_device(dev);/*增加dev中kobject的引用计数*/
if (!dev)
goto done;
if (!dev->p) {/*如果设备对象的私有对象不存在,分配并初始化*/
error = device_private_init(dev);
if (error)
goto done;
}
/*
* for statically allocated devices, which should all be converted
* some day, we need to initialize the name. We prevent reading back
* the name, and force the use of dev_name()
*/
if (dev->init_name) {/*将名称保存到kobj中*/
dev_set_name(dev, "%s", dev->init_name);
dev->init_name = NULL;
}
if (!dev_name(dev))/*从kobj中获取上面设置的名称*/
goto name_error;
pr_debug("device: '%s': %s\n", dev_name(dev), __func__);
/*得到设备的父设备,并增加其引用计数*/
parent = get_device(dev->parent);
/*设置设备的obj对象树,下面详细讲*/
setup_parent(dev, parent);
/* use parent numa_node */
if (parent)
set_dev_node(dev, dev_to_node(parent));
/* first, register with generic layer. */
/* we require the name to be set before, and pass NULL */
/*将obj对象添加到对象树中,对于这里的意思即platform添加到sys目录下*/
error = kobject_add(&dev->kobj, dev->kobj.parent, NULL);
实现效果如下图中的/sys/devices/目录下生成platform文件夹:

if (error)
goto Error;
/* notify platform of device entry */
if (platform_notify)
platform_notify(dev);
/*为文件创建dient对象(其实就是属性),并加入到dient对象树中*/
/*在对应设备下面创建uevent文件*/
error = device_create_file(dev, &uevent_attr);
如下图,在/sys/devices/platform下生成uevent文件

if (error)
goto attrError;
/*如果存在主设备号,在我们的情形中没有主设备号*/
if (MAJOR(dev->devt)) {
/*创建dient对象,用指定的属性,即生成一个dev文件*/
error = device_create_file(dev, &devt_attr);
如果有主设备号,即在dev->kobj下生成dev文件,例如,/sys/devices/virtual/mem/random具有的主设备号为1,次设备号为8,在/sys/devices/virtual/mem/random目录下生成dev文件:

其中dev文件为文本文件,保存内容为major:minor,例子中为1:8:

if (error)
goto ueventattrError;
/*创建/dev符号链接(就是在dient树种创建符号链接树)*/
/*根据设备类型,在sys/dev/char或block或其属性指定的dev_obj目录下生成链接文件,其名字的样式为major:minor,如1:8,指向dev主目录*/
error = device_create_sys_dev_entry(dev);
继续上面的例子,在这里为在/sys/dev/char/下生成1:8/文件夹链接到/sys/devices/virtual/mem/random目录:

if (error)
goto devtattrError;
devtmpfs_create_node(dev);
}
/*添加链接文件,dev的class存在时有效,在后面详细讲*/
error = device_add_class_symlinks(dev);
if (error)
goto SymlinkError;
/*实际负责device中的属性添加。也是几个部分的集合,
包括class中的dev_attrs,device_type中的groups,还有device本
身的groups。*/
error = device_add_attrs(dev);
if (error)
goto AttrsError;
/*add device to bus*/
/*添加device到bus,建立bus和device之间的联系,后面详细将*/
error = bus_add_device(dev);
if (error)
goto BusError;
/*在dev->kobj目录下生成power目录,用于电源管理*/
error = dpm_sysfs_add(dev);
在dev->kobj下会生成一个power文件夹用于电源的管理,例如我们这里会在platform下
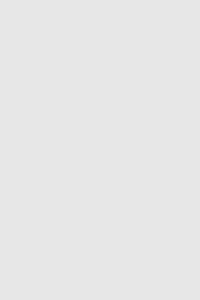
if (error)
goto DPMError;
/*添加设备到PM链表*/
device_pm_add(dev);
/* Notify clients of device addition. This call must come
* after dpm_sysf_add() and before kobject_uevent().
*/
if (dev->bus)
blocking_notifier_call_chain(&dev->bus->p->bus_notifier,
BUS_NOTIFY_ADD_DEVICE, dev);
kobject_uevent(&dev->kobj, KOBJ_ADD);
/*将驱动与设备关联,在后面详细讲解*/
bus_probe_device(dev);
/*下面为添加到系统中的各种链表中*/
if (parent)
klist_add_tail(&dev->p->knode_parent,
&parent->p->klist_children);
if (dev->class) {
mutex_lock(&dev->class->p->class_mutex);
/* tie the class to the device */
klist_add_tail(&dev->knode_class,
&dev->class->p->class_devices);
/* notify any interfaces that the device is here */
list_for_each_entry(class_intf,
&dev->class->p->class_interfaces, node)
if (class_intf->add_dev)
class_intf->add_dev(dev, class_intf);
mutex_unlock(&dev->class->p->class_mutex);
}
……
}
下面为一些辅助函数的实现
- int device_private_init(struct device *dev)
- {
-
- dev->p = kzalloc(sizeof(*dev->p), GFP_KERNEL);
- if (!dev->p)
- return -ENOMEM;
- dev->p->device = dev;
-
-
-
- klist_init(&dev->p->klist_children, klist_children_get,
- klist_children_put);
- return 0;
- }
- static struct kobject *get_device_parent(struct device *dev,
- struct device *parent)
- {
- int retval;
-
- if (dev->class) {
- struct kobject *kobj = NULL;
- struct kobject *parent_kobj;
- struct kobject *k;
-
-
-
-
-
-
- if (parent == NULL)
-
-
-
-
-
- parent_kobj = virtual_device_parent(dev);
- else if (parent->class)
- return &parent->kobj;
- else
- parent_kobj = &parent->kobj;
-
-
- spin_lock(&dev->class->p->class_dirs.list_lock);
- list_for_each_entry(k, &dev->class->p->class_dirs.list, entry)
- if (k->parent == parent_kobj) {
- kobj = kobject_get(k);
- break;
- }
- spin_unlock(&dev->class->p->class_dirs.list_lock);
- if (kobj)
- return kobj;
-
-
-
-
- k = kobject_create();
- if (!k)
- return NULL;
-
- k->kset = &dev->class->p->class_dirs;
-
- retval = kobject_add(k, parent_kobj, "%s", dev->class->name);
- if (retval < 0) {
- kobject_put(k);
- return NULL;
- }
-
- return k;
- }
-
- if (parent)
- return &parent->kobj;
- return NULL;
- }
- static struct kobject *virtual_device_parent(struct device *dev)
- {
- static struct kobject *virtual_dir = NULL;
-
- if (!virtual_dir)
- virtual_dir = kobject_create_and_add("virtual",
- &devices_kset->kobj);
-
- return virtual_dir;
- }
我们看看具体在Linux下的相关目录:
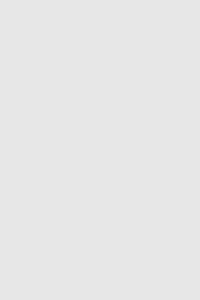
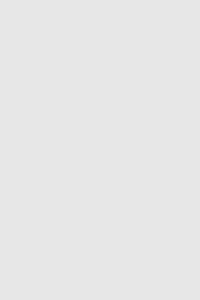
设备与class关联
static int device_add_class_symlinks(struct device *dev)
{
int error;
/*没有class的情况直接返回*/
if (!dev->class)
return 0;
/*在dev->kobj目录下生成一个名为'subsystem'的链接文件,指向其所属的class的sys 的目录/sys/class/***,例如,接着上面的例子
*/
error = sysfs_create_link(&dev->kobj,
&dev->class->p->class_subsys.kobj,
"subsystem");
生成下图:
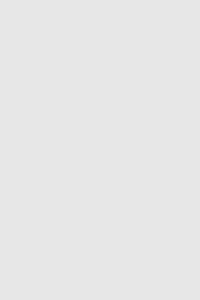
……
}
device关联bus
int bus_add_device(struct device *dev)
{
struct bus_type *bus = bus_get(dev->bus);
int error = 0;
/*如果bus存在,才执行下面部分*/
if (bus) {
pr_debug("bus: '%s': add device %s\n", bus->name, dev_name(dev));
/*添加属性文件,该属性文件问bus的属性*/
error = device_add_attrs(bus, dev);
if (error)
goto out_put;
/*在bus的devices目录下生成到dev->kobj的链接,名称为dev->kobj的名称*/
error = sysfs_create_link(&bus->p->devices_kset->kobj,
&dev->kobj, dev_name(dev));
例如,我们看/sys/devices/platform/devices/下的serial8250,为链接到/sys/devices/platform/serial8250
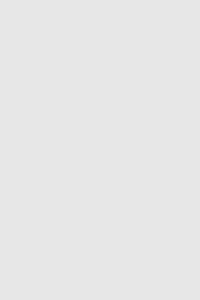
if (error)
goto out_id;
/*dev->kobj目录下subsystem为生成的到bus 下相关
目录的链接*/
error = sysfs_create_link(&dev->kobj,
&dev->bus->p->subsys.kobj, "subsystem");
我们看是考虑前面的serial8250文件,可见其subsystem目录链接到/sys/bus/platform/
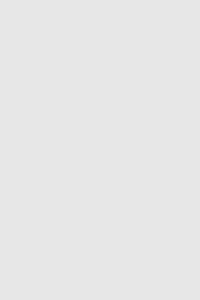
if (error)
goto out_subsys;
/*dev->kobj目录下的bus生成到bus主目录的链接。
在我机器上没有定义相关的宏*/
error = make_deprecated_bus_links(dev);
if (error)
goto out_deprecated;
/*添加bus的设备链表到dev下的bus链表上*/
klist_add_tail(&dev->p->knode_bus, &bus->p->klist_devices);
}
return 0;
……
}
设备与驱动关联:
-
-
-
-
-
-
-
- void bus_probe_device(struct device *dev)
- {
- struct bus_type *bus = dev->bus;
- int ret;
-
- if (bus && bus->p->drivers_autoprobe) {
- ret = device_attach(dev);
- WARN_ON(ret < 0);
- }
- }
Device_attach()函数最终调用driver_sysfs_add()函数实现实际工作
static int driver_sysfs_add(struct device *dev)
{
int ret;
/*驱动目录下dev->kobj目录链接到dev->kobj*/
ret = sysfs_create_link(&dev->driver->p->kobj, &dev->kobj,
kobject_name(&dev->kobj));
我门仍然以serial8250为例子来说明,生成的链接如下图:
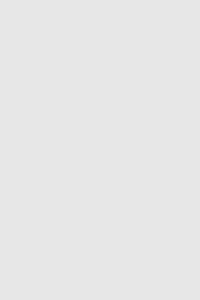
if (ret == 0) {
/*在dev->kobj目录下的driver目录链接到其驱动目录*/
ret = sysfs_create_link(&dev->kobj, &dev->driver->p->kobj,
"driver");
生成链接结果如下图所示:
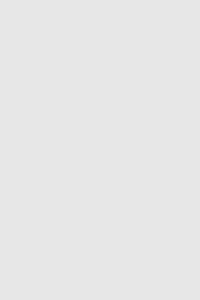
if (ret)
sysfs_remove_link(&dev->driver->p->kobj,
kobject_name(&dev->kobj));
}
return ret;
}
注册bus
- struct bus_type platform_bus_type = {
- .name = "platform",
- .dev_attrs = platform_dev_attrs,
- .match = platform_match,
- .uevent = platform_uevent,
- .pm = &platform_dev_pm_ops,
- };
- int bus_register(struct bus_type *bus)
- {
- int retval;
- struct bus_type_private *priv;
-
- priv = kzalloc(sizeof(struct bus_type_private), GFP_KERNEL);
- if (!priv)
- return -ENOMEM;
-
- priv->bus = bus;
- bus->p = priv;
-
- BLOCKING_INIT_NOTIFIER_HEAD(&priv->bus_notifier);
-
- retval = kobject_set_name(&priv->subsys.kobj, "%s", bus->name);
- if (retval)
- goto out;
-
- priv->subsys.kobj.kset = bus_kset;
- priv->subsys.kobj.ktype = &bus_ktype;
- priv->drivers_autoprobe = 1;
-
- retval = kset_register(&priv->subsys);
- if (retval)
- goto out;
-
- retval = bus_create_file(bus, &bus_attr_uevent);
- if (retval)
- goto bus_uevent_fail;
-
- priv->devices_kset = kset_create_and_add("devices", NULL,
- &priv->subsys.kobj);
- if (!priv->devices_kset) {
- retval = -ENOMEM;
- goto bus_devices_fail;
- }
-
- priv->drivers_kset = kset_create_and_add("drivers", NULL,
- &priv->subsys.kobj);
- if (!priv->drivers_kset) {
- retval = -ENOMEM;
- goto bus_drivers_fail;
- }
-
- klist_init(&priv->klist_devices, klist_devices_get, klist_devices_put);
- klist_init(&priv->klist_drivers, NULL, NULL);
-
-
- retval = add_probe_files(bus);
- if (retval)
- goto bus_probe_files_fail;
-
- retval = bus_add_attrs(bus);
- if (retval)
- goto bus_attrs_fail;
-
- pr_debug("bus: '%s': registered\n", bus->name);
- return 0;
-
- bus_attrs_fail:
- remove_probe_files(bus);
- bus_probe_files_fail:
- kset_unregister(bus->p->drivers_kset);
- bus_drivers_fail:
- kset_unregister(bus->p->devices_kset);
- bus_devices_fail:
- bus_remove_file(bus, &bus_attr_uevent);
- bus_uevent_fail:
- kset_unregister(&bus->p->subsys);
- kfree(bus->p);
- out:
- bus->p = NULL;
- return retval;
- }
实现结果如下图,在/sys/bus/platform目录下生成drivers和devices两个文件夹,uevent、drivers_probe、drivers_autoprobe三个属性
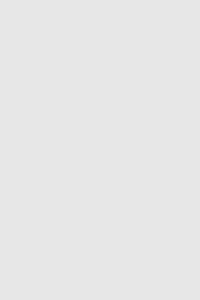
初始化system_bus,实现结果为在/sys/devices/下创建system文件夹
- int __init system_bus_init(void)
- {
- system_kset = kset_create_and_add("system", NULL, &devices_kset->kobj);
- if (!system_kset)
- return -ENOMEM;
- return 0;
- }
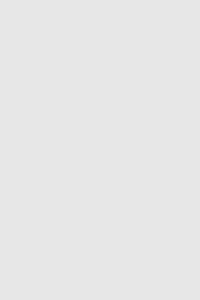
cpu_dev初始化
- int __init cpu_dev_init(void)
- {
- int err;
-
- err = sysdev_class_register(&cpu_sysdev_class);
- if (!err)
- err = cpu_states_init();
-
- #if defined(CONFIG_SCHED_MC) || defined(CONFIG_SCHED_SMT)
- if (!err)
- err = sched_create_sysfs_power_savings_entries(&cpu_sysdev_class);
- #endif
-
- return err;
- }
实现结果如下图,在/sys/devices/system下生成cpu文件夹
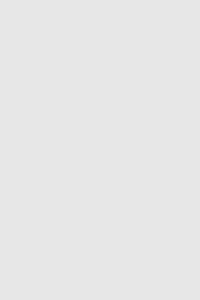
分析了内核怎样对设备驱动模型初始化后对驱动模型的理解应该有一个宏观的概念了,涉及到实际驱动的编写,每种设备有各自的一套机制,但原理上都大同小异。
欢迎转载,转载请注明出处