1. 事件冒泡

阻止事件冒泡的两种方式:
- event.stopPropagation();
- return false ;
2. 绑定事件——bind(type,[data],function)
type为一个或多个事件类型的字符串,data是作为event.data属性值传递给事件对象的额外对象。
$("#btn").bind( "click mouseover", function () { ...);
1
2
3
4
5
6
7
8
9
10
11
12
|
$( function () {
$( ".txt" ).bind({
"focus" : function () {
$( "#divTip" ).html( "请输入" ).show();
},
"blur" : function () {
$( "#divTip" )
.show()
.html( "合法" );
}
});
});
|
var info = { name: 'Cathy', date: '2014-1-24' };
$(function () {
$("#test").bind("click", info, function (event) {
$("#divTip").show().html(event.data.name + "," + event.data.date);
});
});
3.事件切换
$(function () {
$(".clsTitle").hover(
function () {
$(".clsContent").show();
},
function () {
$(".clsContent").hide();
});
});
- toggle:依次顺序调用N个函数,最后一个调用完成后再从第一个轮流执行。
$(function () {
$("#divTest").toggle(
function () {
alert(1);
},
function () {
alert(2);
},
function () {
alert(3);
}
);
});
4.移除事件——unbind(type,func)
参数说明:type为要移除的事件类型,func为要移除的事件处理函数。如果func为空,则移除元素所有的事件。
function func() {
$("#divTip").append("点击按钮2");
}
$(function () {
$("#Button1").click(function () {
$("#divTip").append("点击按钮1");
});
$("#Button2").click(func);
$("#Button3").click(function () {
$("input").unbind("click", func);
});
});
5.其他事件
one(type,[data],func)——为元素绑定只执行一次的事件。
trigger(type,[data])——在所选择的元素上触发指定类型的事件。
$(function () {
var i = 1;
function btn_Click() {
this.value = i++;
}
$( "input").one("click" , btn_Click);
$( "input").bind("click" , btn_Click);
$( "input").trigger("click" );
})
6.实例应用
①选项卡效果
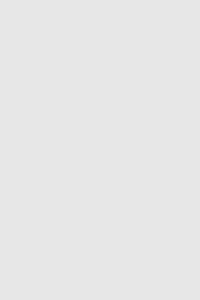
<body>
<ul id="menu">
<li class="tabFocus">家居 </li>
<li> 电器</li >
<li> 二手</li >
</ul>
<ul id="content">
<li class="conFocus">我是家居的内容 </li>
<li> 欢迎您来到电器城 </li>
<li> 二手市场,产品丰富多彩 </li>
</ul>
</body>
html Body
<script type="text/javascript">
$( function () {
$( "#menu li").each(function (index) {
$( this).click(function () {
$( this).addClass("tabFocus" ).siblings().removeClass("tabFocus");
$( "#content li:eq(" + index + ")" ).show().siblings().hide();
});
});
});
</script>
②屏幕中间弹窗遮罩
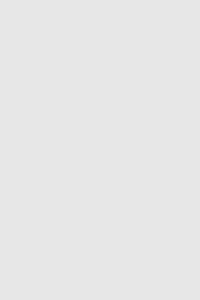
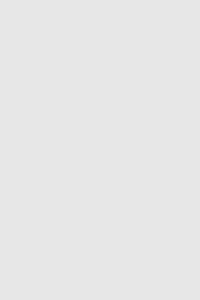
<style type="text/css">
body {
font-size: 13px;
}
.divShow {
line-height: 32px;
height: 32px;
background-color: #eee;
width: 280px;
padding-left: 10px;
}
.divShow span {
padding-left: 50px;
}
.dialog {
width: 360px;
border: solid 5px #666;
position: absolute;
display: none;
z-index: 101;
}
.dialog .title {
background-color: #fbaf15;
padding: 10px;
color: #fff;
font-weight: bold;
}
.dialog .title img {
float: right;
}
.dialog .content {
background-color: #fff;
padding: 25px;
height: 60px;
}
.dialog .content img {
float: left;
}
.dialog .content span {
float: left;
padding-top: 10px;
padding-left: 10px;
}
.dialog .bottom {
text-align: right;
padding: 10px 10px 10px 0px;
background-color: #eee;
}
.mask {
width: 100%;
height: 100%;
background-color: #000;
position: absolute;
top: 0px;
left: 0px;
filter: alpha(opacity=30);
display: none;
z-index: 100;
}
.btn {
border: #666 1px solid;
padding: 2px;
width: 65px;
filter: progid:DXImageTransform.Microsoft.Gradient(GradientType=0,StartColorStr=#ffffff, EndColorStr=#ECE9D8);
}
</style>
<div class="divShow">
<input id="Checkbox1" type="checkbox" />
<a href="#">这是一条可删除的记录</a>
<span>
<input id="Button1" type="button" value="删除" class="btn" />
</span>
</div>
<div class="mask"></div>
<div class="dialog">
<div class="title">
<img src="Images/close.gif" alt="点击可以关闭" />删除时提示
</div>
<div class="content">
<img src="Images/delete.jpg" alt="" /><span>您真的要删除该条记录吗?</span>
</div>
<div class="bottom">
<input id="Button2" type="button" value="确定" class="btn"/>
<input id="Button3" type="button" value="取消" class="btn"/>
</div>
</div>
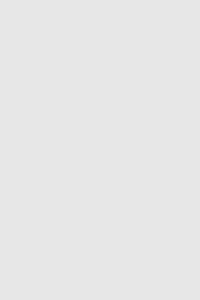
html
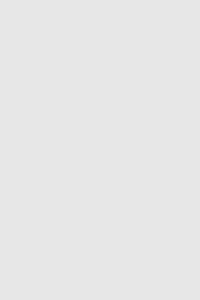
<script type="text/javascript">
$(function () {
$("#Button1").click(function () { //注册删除按钮点击事件
$(".mask").show(); //显示背景色
showDialog(); //设置提示对话框的Top与Left
$(".dialog").show(); //显示提示对话框
})
/*
*根据当前页面与滚动条位置,设置提示对话框的Top与Left
*/
function showDialog() {
var objW = $(window); //当前窗口
var objC = $(".dialog"); //对话框
var brsW = objW.width();
var brsH = objW.height();
var sclL = objW.scrollLeft();
var sclT = objW.scrollTop();
var curW = objC.width();
var curH = objC.height();
//计算对话框居中时的左边距
var left = sclL + (brsW - curW) / 2;
//计算对话框居中时的上边距
var top = sclT + (brsH - curH) / 2;
//设置对话框在页面中的位置
objC.css({ "left": left, "top": top });
}
$(window).resize(function () {//页面窗口大小改变事件
if (!$(".dialog").is(":visible")) {
return;
}
showDialog(); //设置提示对话框的Top与Left
});
$(".title img").click(function () { //注册关闭图片点击事件
$(".dialog").hide();
$(".mask").hide();
})
$("#Button3").click(function () {//注册取消按钮点击事件
$(".dialog").hide();
$(".mask").hide();
})
$("#Button2").click(function () {//注册确定按钮点击事件
$(".dialog").hide();
$(".mask").hide();
if ($("input:checked").length != 0) {//如果选择了删除行
$(".divShow").remove(); //删除某行数据
}
})
})
</script>
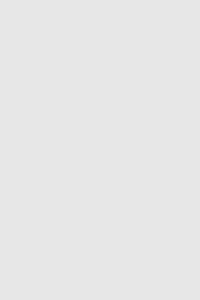