今天的工作
1、append() 方法
Html5代码  - <!DOCTYPE html>
- <html>
- <head>
- <script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js">
- </script>
- <script>
- $(document).ready(function(){
- $("#btn1").click(function(){
- $("p").append(" <b>Appended text</b>.");
- });
-
- $("#btn2").click(function(){
- $("ol").append("<li>Appended item</li>");
- });
- });
- </script>
- </head>
-
- <body>
- <p>This is a paragraph.</p>
- <p>This is another paragraph.</p>
- <ol>
- <li>List item 1</li>
- <li>List item 2</li>
- <li>List item 3</li>
- </ol>
- <button id="btn1">追加文本</button>
- <button id="btn2">追加列表项</button>
- </body>
- </html>
append() 方法可以将原本没有的html通过jquery加进去只要使用和前面同样的class就可以在添加的瞬间完成style的设置
2、prepend() 方法
Html5代码  - <!DOCTYPE html>
- <html>
- <head>
- <script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
- <script>
- $(document).ready(function(){
- $("#btn1").click(function(){
- $("p").prepend("<b>Prepended text</b>. ");
- });
- $("#btn2").click(function(){
- $("ol").prepend("<li>Prepended item</li>");
- });
- });
- </script>
- </head>
- <body>
-
- <p>This is a paragraph.</p>
- <p>This is another paragraph.</p>
- <ol>
- <li>List item 1</li>
- <li>List item 2</li>
- <li>List item 3</li>
- </ol>
-
- <button id="btn1">添加文本</button>
- <button id="btn2">添加列表项</button>
-
- </body>
- </html>
prepend() 方法跟前面的元素添加是一样的效果但是一样的在于在面的方法是在默认的到最后进行添加但是prepend() 方法是可以在最前面开始进行添加
3、remove() 方法
Html5代码  - <!DOCTYPE html>
- <html>
- <head>
- <script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
- <script>
- $(document).ready(function(){
- $("button").click(function(){
- $("#div1").remove();
- });
- });
- </script>
- </head>
-
- <body>
-
- <div id="div1" style="height:100px;width:300px;border:1px solid black;background-color:yellow;">
- This is some text in the div.
- <p>This is a paragraph in the div.</p>
- <p>This is another paragraph in the div.</p>
- </div>
-
- <br>
- <button>删除 div 元素</button>
-
- </body>
- </html>
remove() 方法可以把指定的元素通过remove() 方法给删除掉在那里面就是把整个div给全部删除掉
4、empty() 方法
Html5代码  - <!DOCTYPE html>
- <html>
- <head>
- <script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
- <script>
- $(document).ready(function(){
- $("button").click(function(){
- $("#div1").empty();
- });
- });
- </script>
- </head>
-
- <body>
-
- <div id="div1" style="height:100px;width:300px;border:1px solid black;background-color:yellow;">
- This is some text in the div.
- <p>This is a paragraph in the div.</p>
- <p>This is another paragraph in the div.</p>
- </div>
-
- <br>
- <button>清空 div 元素</button>
-
- </body>
- </html>
empty() 方法同样是删除但是不一样的事前者是全部删除连div自身一起删除但是empty() 方法不一样empty() 方法是清空意思就是可以把指定的div里面的内容全部清空但是会保留下当前的div
5、addClass() 方法
Html5代码  - <!DOCTYPE html>
- <html>
- <head>
- <script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js">
- </script>
- <script>
- $(document).ready(function(){
- $("button").click(function(){
- $("#div1").addClass("important blue");
- });
- });
- </script>
- <style type="text/css">
- .important
- {
- font-weight:bold;
- font-size:xx-large;
- }
- .blue
- {
- color:blue;
- }
- </style>
- </head>
- <body>
-
- <div id="div1">这是一些文本。</div>
- <div id="div2">这是一些文本。</div>
- <br>
- <button>向第一个 div 元素添加类</button>
-
- </body>
- </html>
addClass() 方法是可以通过jquery直接向一个没有class的标签里面添加进入一个带有属性的class在里面在点击按钮以后原来的第二行文字会发生变化对应$("#div1").addClass("important blue");这条语句的意思是变成蓝色加大
6、removeClass() 方法
Html5代码 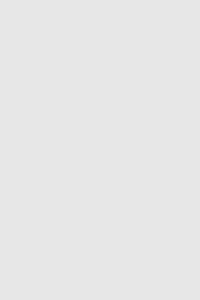 - <!DOCTYPE html>
- <html>
- <head>
- <script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
- <script>
- $(document).ready(function(){
- $("button").click(function(){
- $("h1,h2,p").removeClass("blue");
- });
- });
- </script>
- <style type="text/css">
- .important
- {
- font-weight:bold;
- font-size:xx-large;
- }
- .blue
- {
- color:blue;
- }
- </style>
- </head>
- <body>
-
- <h1 class="blue">标题 1</h1>
- <h2 class="blue">标题 2</h2>
- <p class="blue">这是一个段落。</p>
- <p>这是另一个段落。</p>
- <br>
- <button>从元素上删除类</button>
- </body>
- </html>
removeClass() 方法是可以将原本字体的蓝色给删除掉不是改变是删除掉
7、toggleClass() 方法
Html5代码 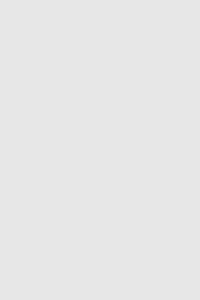 - <!DOCTYPE html>
- <html>
- <head>
- <script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js">
- </script>
- <script>
- $(document).ready(function(){
- $("button").click(function(){
- $("h1,h2,p").toggleClass("blue");
- });
- });
- </script>
- <style type="text/css">
- .blue
- {
- color:blue;
- }
- </style>
- </head>
- <body>
-
- <h1>标题 1</h1>
- <h2>标题 2</h2>
- <p>这是一个段落。</p>
- <p>这是另一个段落。</p>
- <button>切换 CSS 类</button>
- </body>
- </html>
toggleClass() 方法有着上面两种效果在没有蓝色的字体上加上蓝色在有蓝色的字体上清除蓝色
|