命名纹理对象:
- void glGenTextures (GLsizei n, GLuint *textures);
- //在数组textures中返回n个当期未使用的值,表示纹理对象的名称
- //零作为一个保留的纹理对象名,它不会被此函数当做纹理对象名称而返回
判断一个纹理名称是否处于实际使用中:
- GLboolean glIsTexture (GLuint texture);
- //如果texture是一个已绑定的纹理对象名称,并且没有删除,就返回GL_TRUE;
创建和使用纹理对象:
- glBindTexture(GL_TEXTURE_2D, texName[0]);
- void glBindTexture (GLenum target, GLuint texture);
- //应用程序第一次在这个函数使用texture值时,这个函数将会创建一个新的纹理对象
- //当texture是一个以前已经创建的纹理对象时,这个纹理对象就成为活动对象
- //如果texture为0,就停止使用这个纹理对象,并返回无名称的默认纹理
清除纹理对象:
- glDeleteTextures(2, texName);
- void glDeleteTextures (GLsizei n, const GLuint *textures);
- //删除n个纹理对象,它们的名称由textures数组提供
实例:
- #include <GL/glut.h>
- #include <stdlib.h>
- #include <stdio.h>
-
- #ifdef GL_VERSION_1_1
- /* Create checkerboard texture */
- #define checkImageWidth 64
- #define checkImageHeight 64
- static GLubyte checkImage[checkImageHeight][checkImageWidth][4];
- static GLubyte otherImage[checkImageHeight][checkImageWidth][4];
-
- static GLuint texName[2];
-
- void makeCheckImages(void)
- {
- int i, j, c;
-
- for (i = 0; i < checkImageHeight; i++) {
- for (j = 0; j < checkImageWidth; j++) {
- c = ((((i&0x8)==0)^((j&0x8))==0))*255;
- checkImage[i][j][0] = (GLubyte) c;
- checkImage[i][j][1] = (GLubyte) c;
- checkImage[i][j][2] = (GLubyte) c;
- checkImage[i][j][3] = (GLubyte) 255;
- c = ((((i&0x10)==0)^((j&0x10))==0))*255;
- otherImage[i][j][0] = (GLubyte) c;
- otherImage[i][j][1] = (GLubyte) 0;
- otherImage[i][j][2] = (GLubyte) 0;
- otherImage[i][j][3] = (GLubyte) 255;
- }
- }
- }
-
- void init(void)
- {
- glClearColor (0.0, 0.0, 0.0, 0.0);
- glShadeModel(GL_FLAT);
- glEnable(GL_DEPTH_TEST);
-
- makeCheckImages();
- glPixelStorei(GL_UNPACK_ALIGNMENT, 1);
-
- glGenTextures(2, texName);
- glBindTexture(GL_TEXTURE_2D, texName[0]);
- glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP);
- glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP);
- glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER,
- GL_NEAREST);
- glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER,
- GL_NEAREST);
- glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, checkImageWidth,
- checkImageHeight, 0, GL_RGBA, GL_UNSIGNED_BYTE,
- checkImage);
-
- glBindTexture(GL_TEXTURE_2D, texName[1]);
- glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP);
- glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP);
- glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST);
- glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST);
- glTexEnvf(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_DECAL);
- glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, checkImageWidth,
- checkImageHeight, 0, GL_RGBA, GL_UNSIGNED_BYTE,
- otherImage);
-
- glEnable(GL_TEXTURE_2D);
- }
-
- void display(void)
- {
- glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
- glBindTexture(GL_TEXTURE_2D, texName[0]);
- glBegin(GL_QUADS);
- glTexCoord2f(0.0, 0.0); glVertex3f(-2.0, -1.0, 0.0);
- glTexCoord2f(0.0, 1.0); glVertex3f(-2.0, 1.0, 0.0);
- glTexCoord2f(1.0, 1.0); glVertex3f(0.0, 1.0, 0.0);
- glTexCoord2f(1.0, 0.0); glVertex3f(0.0, -1.0, 0.0);
- glEnd();
- glBindTexture(GL_TEXTURE_2D, texName[1]);
- glBegin(GL_QUADS);
- glTexCoord2f(0.0, 0.0); glVertex3f(1.0, -1.0, 0.0);
- glTexCoord2f(0.0, 1.0); glVertex3f(1.0, 1.0, 0.0);
- glTexCoord2f(1.0, 1.0); glVertex3f(2.41421, 1.0, -1.41421);
- glTexCoord2f(1.0, 0.0); glVertex3f(2.41421, -1.0, -1.41421);
- glEnd();
- glFlush();
- }
-
- void reshape(int w, int h)
- {
- glViewport(0, 0, (GLsizei) w, (GLsizei) h);
- glMatrixMode(GL_PROJECTION);
- glLoadIdentity();
- gluPerspective(60.0, (GLfloat) w/(GLfloat) h, 1.0, 30.0);
- glMatrixMode(GL_MODELVIEW);
- glLoadIdentity();
- glTranslatef(0.0, 0.0, -3.6);
- }
-
- void keyboard(unsigned char key, int x, int y)
- {
- switch (key) {
- case 27:
- exit(0);
- break;
- }
- }
-
- int main(int argc, char** argv)
- {
- glutInit(&argc, argv);
- glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB | GLUT_DEPTH);
- glutInitWindowSize(250, 250);
- glutInitWindowPosition(100, 100);
- glutCreateWindow(argv[0]);
- init();
- glutReshapeFunc(reshape);
- glutDisplayFunc(display);
- glutKeyboardFunc (keyboard);
- glutMainLoop();
- return 0;
- }
- #else
- int main(int argc, char** argv)
- {
- fprintf (stderr, "This program demonstrates a feature which is not in OpenGL Version 1.0.\n");
- fprintf (stderr, "If your implementation of OpenGL Version 1.0 has the right extensions,\n");
- fprintf (stderr, "you may be able to modify this program to make it run.\n");
- return 0;
- }
- #endif
运行结果:
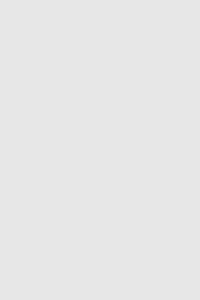
|