poi是java的api用来操作微软的文档
poi的jar包和功能映射如下:

本文是以poi3.9读写2010word、2010excel、2010ppt,记录学习的脚步
相应的功能在代码都有注释,就不解释了 详情可以参看poi3.9的文档
主测试函数 TestMain.java
- /**
- *
- */
- package com.undergrowth.poi.test;
-
- import com.undergrowth.poi.ExcelUtils;
- import com.undergrowth.poi.PptUtils;
- import com.undergrowth.poi.WordUtils;
-
- /**
- * @author u1
- *
- */
- public class TestMain {
-
- /**
- * 1.对于word,使用XWPFDocument操作07以上的doc或者docx都没有问题,并且必须是07或者以上的电脑上生成的word
- * 如果是WordExtractor或者HWPFDocument只能操作03以下的word,并且只能是03以下电脑生成的word
- *
- * @param args
- */
- public static void main(String[] args) {
- // TODO Auto-generated method stub
- String path="e:\\poi\\";
- String fileName="poi.docx";
- String filePath=path+fileName;
- //创建word
- //WordUtils.createWord(path,fileName);
- //写入数据
- String data="两国元首在亲切友好、相互信任的气氛中,就中乌关系现状和发展前景,以及共同关心的国际和地区问题深入交换了意见,达成广泛共识。两国元首高度评价中乌关系发展成果,指出建立和发展战略伙伴关系是正确的历史选择,拓展和深化双方各领域合作具有广阔前景和巨大潜力,符合两国和两国人民的根本利益。";
-
- WordUtils.writeDataDocx(filePath,data);
- //WordUtils.writeDataDoc(filePath,data);
-
- //读取数据
- //String contentWord=WordUtils.readDataDoc(filePath);
- String contentWord=WordUtils.readDataDocx(filePath);
- System.out.println("word的内容为:\n"+contentWord);
-
- //创建excel
- fileName="poi.xlsx";
- filePath=path+fileName;
- String[] unitTitle={"google","baidu","oracle","合计"};
- String[] itemTitle={"单位","总收入","盈利","亏损"};
- String[] dataExcel={"10","20","30"};
- String title="各大公司收入情况";
- ExcelUtils.writeDataExcelx(filePath,dataExcel,title,unitTitle,itemTitle);
-
- //读取数据
- String contentExcel=ExcelUtils.readDataExcelx(filePath);
- System.out.println("\nexcel的内容为:\n"+contentExcel);
-
- //创建ppt
- fileName="poi.pptx";
- filePath=path+fileName;
- String titlePptx="华尔街纪录片";
- String imagePath="images/hej.jpg";
- PptUtils.writeDataPptx(filePath,titlePptx,imagePath);
-
- //读取pptx的数据
- String contentPptx=PptUtils.readDataPptx(filePath);
- System.out.println("\nppt的内容为:\n"+contentPptx);
- }
-
- }
word操作工具 WordUtils.java
excel工具 ExcelUtils.java
ppt的工具 PptUtils.java
项目结构图:

效果图:
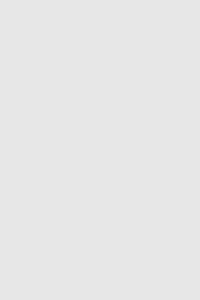
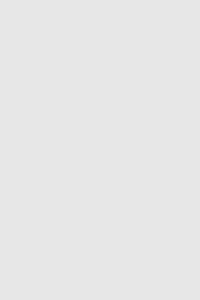
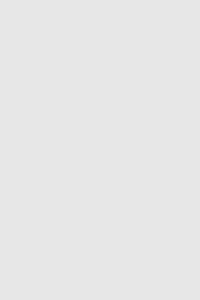
控制台输出:
- 创建word成功
- word的内容为:
- 两国元首在亲切友好、相互信任的气氛中,就中乌关系现状和发展前景,以及共同关心的国际和地区问题深入交换了意见,达成广泛共识。两国元首高度评价中乌关系发展成果,指出建立和发展战略伙伴关系是正确的历史选择,拓展和深化双方各领域合作具有广阔前景和巨大潜力,符合两国和两国人民的根本利益。
- 创建excel成功
-
- excel的内容为:
- 各大公司收入情况
- 单位 总收入 盈利 亏损
- google 10.0 20.0 30.0
- baidu 10.0 20.0 30.0
- oracle 10.0 20.0 30.0
- 合计 sum(b2:b5) sum(c2:c5) sum(d2:d5)
-
- 创建pptx成功
-
- ppt的内容为:
-
- 华尔街纪录片
- 华尔街纪录片 华尔街是纽约市曼哈顿区南部从百老汇路延伸到东河的一条大街道的名字 “华尔街”一词现已超越这条街道本身,成为附近区域的代称,亦可指对整个美国经济具有影响力的金融市场和金融机构。
|