随着Web前端技术的迅猛发展,现在的Web开发已经明显分为两大阵营:Web前端和Web后端,接着UI设计又从Web前端分离出去,成为专门的团队。当我们从JavaScript开始,一路经过jQuery、Bootstrap、React,一直追逐到Vue时,是否还有人记得曾经的Web开发是前后端一体的?
本文给出的前后端一体的Web服务器例子,这种开发方式绝对不值得提倡,但是这个例子,将有助于我们加深理解HTTP和HTML。
1、需求定义
本次开发,要求在网页上显示某个培训班的所有学生信息。
我们用MySQL保存学生信息,使用SpringBoot构建程序框架,使用MyBatis与数据库对接。
下面是详细开发过程。
2、在MySQL服务器上执行下面的SQL语句创建db_train数据库,在db_train库中建立t_student表:
create database db_train default character set utf8;use db_train;create table t_student(
student_id int unsigned not null,
student_name varchar(32) not null,
student_age int unsigned not null,
student_gender int not null,
primary key(student_id)
)engine=InnoDB default charset=utf8;
3、使用下面的SQL语句向t_student表中插入一些实验数据:
insert into t_student(student_id, student_name, student_age, student_gender) values(1, '张三', 36, 1);insert into t_student(student_id, student_name, student_age, student_gender) values(2, '李明', 31, 0);insert into t_student(student_id, student_name, student_age, student_gender) values(3, '王麻', 28, 0);insert into t_student(student_id, student_name, student_age, student_gender) values(4, '郑丽', 27, 1);insert into t_student(student_id, student_name, student_age, student_gender) values(5, '赵进', 39, 0);insert into t_student(student_id, student_name, student_age, student_gender) values(6, '郭霞', 33, 1);insert into t_student(student_id, student_name, student_age, student_gender) values(7, '杨浩', 37, 0);
4、查询数据库,确认上面的SQL语句执行已经成功:
5、启动IDEA,创建一个空的工程train:
6、在工程中创建一个SpringBoot模块student,Group输入com.flying,Java版本选择8,Dependencies选择Lombok、SpringWeb、MyBatis Framework:
7、修改pom.xml文件,加入mysql数据库连接的依赖,以及打包生成jar文件的内容。修改后的pom.xml文件为:
<?xml version='1.0' encoding='UTF-8'?><project xmlns='http://maven./POM/4.0.0' xmlns:xsi='http://www./2001/XMLSchema-instance'
xsi:schemaLocation='http://maven./POM/4.0.0 https://maven./xsd/maven-4.0.0.xsd'>
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.3.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.flying</groupId>
<artifactId>student</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>student</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies><dependency><groupId>mysql</groupId><artifactId>mysql-connector-java</artifactId><scope>runtime</scope></dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.3</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
<build>
<finalName>student</finalName>
<plugins>
<!--打包jar-->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<configuration>
<!--不打包资源文件,exclude的目录不是src下面的,是以编译结果classes为根目录计算-->
<excludes>
<exclude>*.properties</exclude>
<exclude>*.txt</exclude>
<exclude>*.xml</exclude>
</excludes>
<archive>
<manifest>
<addClasspath>true</addClasspath>
<!--MANIFEST.MF 中 Class-Path 加入前缀-->
<classpathPrefix>student_lib/</classpathPrefix>
<!--jar包不包含唯一版本标识-->
<useUniqueVersions>false</useUniqueVersions>
<!--指定入口类-->
<mainClass>com.flying.student.StudentApplication</mainClass>
</manifest>
<manifestEntries>
<!--MANIFEST.MF 中 Class-Path 加入资源文件目录-->
<Class-Path>./resources/</Class-Path>
</manifestEntries>
</archive>
<outputDirectory>${project.build.directory}</outputDirectory>
</configuration>
</plugin>
<!--拷贝依赖 copy-dependencies-->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<executions>
<execution>
<id>copy-dependencies</id>
<phase>package</phase>
<goals>
<goal>copy-dependencies</goal>
</goals>
<configuration>
<outputDirectory>
${project.build.directory}/student_lib/ </outputDirectory>
</configuration>
</execution>
</executions>
</plugin>
<!--拷贝资源文件 copy-resources-->
<plugin>
<artifactId>maven-resources-plugin</artifactId>
<executions>
<execution>
<id>copy-resources</id>
<phase>package</phase>
<goals>
<goal>copy-resources</goal>
</goals>
<configuration>
<resources>
<resource>
<directory>src/main/resources</directory>
</resource>
</resources>
<outputDirectory>${project.build.directory}/resources</outputDirectory>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build></project>
8、在src\main\java\com\flying\student目录中增加entity包,然后在entity包中增加StudentEntity数据类,代码如下:
package com.flying.student.entity;import lombok.Data;@Datapublic class StudentEntity { private int studentId; private String studentName; private int studentAge; private int studentGender; }
9、在src\main\java\com\flying\student目录中增加dao包,然后在dao包中增加数据据库操作接口StudentDao,代码如下:
package com.flying.student.dao;import com.flying.student.entity.StudentEntity;import org.apache.ibatis.annotations.Mapper;import java.util.List;@Mapperpublic interface StudentDao { List<StudentEntity> queryAllStudents();
}
10、在src\main\resources目录中增加mapper子目录,然后在mapper子目录中增加StudentMapper.xml文件,文件内容如下:
<?xml version='1.0' encoding='UTF-8' ?><!DOCTYPE mapper PUBLIC '-////DTD Mapper 3.0//EN' 'http:///dtd/mybatis-3-mapper.dtd'><mapper namespace='com.flying.student.dao.StudentDao'> <!-- 查询所有的学生信息 --> <select id='queryAllStudents' resultType='com.flying.student.entity.StudentEntity'> select student_id as studentId, student_name as studentName, student_age as studentAge, student_gender as studentGender from t_student </select></mapper>
11、在src\main\java\com\flying\student目录中增加controller包,然后在controller包中增加StudentController类,代码如下:
package com.flying.student.controller;import com.flying.student.dao.StudentDao;import com.flying.student.entity.StudentEntity;import org.springframework.beans.factory.annotation.Autowired;import org.springframework.stereotype.Controller;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.ResponseBody;import java.util.List;
@Controller
public class StudentController {
@Autowired
private StudentDao studentDao;
@RequestMapping('/all_students')
public @ResponseBody String getAllStudents(){
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append('<html>');
stringBuffer.append(' <head>');
stringBuffer.append(' <title>培训学生信息查询</title>');
stringBuffer.append(' </head>');
stringBuffer.append(' <body>');
stringBuffer.append(' <table align=\'center\' width=\'1000\' border=\'1\'>');
stringBuffer.append(' <tr>');
stringBuffer.append(' <td align=\'center\' colspan=\'4\'>');
stringBuffer.append(' <h2>节点信息查询</h2>');
stringBuffer.append(' </td>');
stringBuffer.append(' </tr>');
stringBuffer.append(' <tr align=\'center\'>');
stringBuffer.append(' <td>学生ID</td>');
stringBuffer.append(' <td>学生姓名</td>');
stringBuffer.append(' <td>学生年龄</td>');
stringBuffer.append(' <td>学生性别</td>');
stringBuffer.append(' </tr>');
List<StudentEntity> nodeEntityList = studentDao.queryAllStudents(); int recordCount; if (nodeEntityList == null){
recordCount = 0;
}else{
recordCount = nodeEntityList.size();
} for (int i=0; i<recordCount; i ){
StudentEntity studentEntity = nodeEntityList.get(i);
stringBuffer.append(' <tr align=\'center\'>');
stringBuffer.append(' <td>').append(studentEntity.getStudentId()).append('</td>');
stringBuffer.append(' <td>').append(studentEntity.getStudentName()).append('</td>');
stringBuffer.append(' <td>').append(studentEntity.getStudentAge()).append('</td>'); if (studentEntity.getStudentGender() == 0){
stringBuffer.append(' <td>男</td>');
}else{
stringBuffer.append(' <td>女</td>');
}
}
stringBuffer.append(' </body>');
stringBuffer.append('</html>'); return stringBuffer.toString();
}
}
12、修改application.properties文件,设置服务器监听端口为8000,设置MySQL和MyBatis的信息:
server.port=8000spring.datasource.url=jdbc:mysql://127.0.0.1/db_train?useUnicode=true&characterEncoding=utf-8&allowMultiQueries=truespring.datasource.username=rootspring.datasource.password=123456spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driverspring.datasource.max-idle=10spring.datasource.max-wait=10000spring.datasource.min-idle=5spring.datasource.initial-size=5mybatis.typeAliasesPackage=spring.SpringBoot.mappermybatis.mapperLocations=classpath:mapper/*.xml
13、在IDEA的Terminal窗口执行 mvn clean package -DskipTests 命令,进行编译和打包:
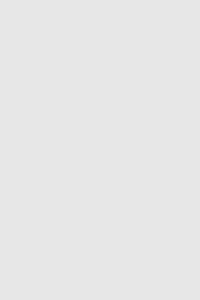
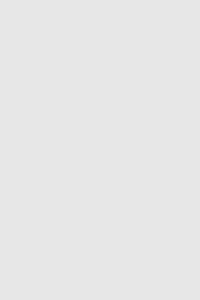
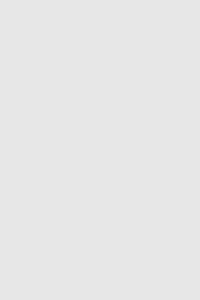
14、编译后将会生成resources目录、student_lib目录和student.jar文件,将这些文件拷贝到Linux运行环境(我比较喜欢Linux环境,其实Windows环境也是可以的哈):
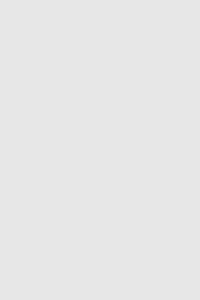
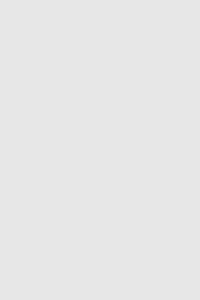
15、在Linux环境下执行生成的student.jar文件:
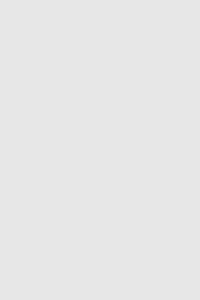
16、打开浏览器,输入 http://<服务器IP地址>:8000/all_students
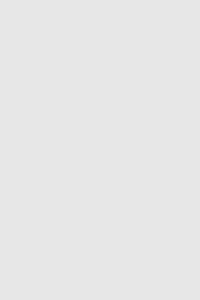
可以看到,网页上以表格形式显示了培训班的所有学生信息,我们通过前后端一体的方式,实现了数据库表内容查询的功能。
谢谢阅读!欢迎收藏。